- 2021-12-1
- best seaside towns uk 2021
Other than Insertion and Deletion, a linked list supports basic functions such as Display, Search, and Delete. Mastering Data Structures & Algorithms using C and C++ for those who are good at C/C++ Data Structures in Java: An Interview Refresher to refresh important Data Structure and algorithms concepts . Use minimum graphics in snakes and ladders as far as possible avoiding complex codes. Full PDF Package Download Full PDF Package. So, here name, address and phone number are those different types of data. Tries in Word Games. In C++, a structure is a user-defined data type. They indeed find it relatively easy to some standard programs like, for example, arrays and pointers, file handling and data structure, functions, etc. An aerial view of boxes with addresses, each containing people & various items, shows neighborhoods connected by their roads. It is a well-known fact that most beginners are going into the world of programming these days to learn C++ as their first programming language. In 95% of cases, you're better off using a standard container in the language you're using (a hashmap, an array list, a linked list, whatever). . Compatible as multi-player game. Hope you all found these C++ implementations of arrays, linked lists, stacks and queues useful. This second edition of Data Structures Using C has been developed to provide a comprehensive and consistent coverage of both the abstract concepts of data structures as well as the implementation of these concepts using C language. It is prohibited to use loops and while(If you do not know what it is do not worry). Studying the concrete array data structure is a great starting point to understanding other data structures. This project is a version of the classical snake game arcade that I developed to practice, and improve, my skills in POO, data structures, file manipulation, C++, and its STL. Compatible as multi-player game. It follows the WORA principle. The best example is trees and graphs. The structure creates a data type for grouping items of different data types under a single data type. A two-dimensional array would be ideal. Viewed up close, you may say that we have a hash table. C/C++ arrays allow you to define variables that combine several data items of the same kind, but structure is another user defined data type which allows you to combine data items of different kinds. I'm doing a Uno Card game (in C) and I have a Circular Queue in this following order: user -> player1 -> player2 -> player3. The point (6, 3) Doubly Linked List Sample in C Language. The fruit is represented with an * (asterisk) symbol. C++ Fast Track for Games Programming Part 13: Data Structures You are quite far into the C++ Fast Track preparation for Games Programming tutorial series. ARCHERY GAME. Data Structure programs using C and C++ (Solved data structure programs) Data Structure Examples / Programs using C and C++ - This section contains solved programs using C and C++ on Data Structure concepts like Sorting (Bubble Sort, Insertion Sort, Selection Sort), Searching (Linear/sequential Search, Binary Search), Stack Implementation using Array, Linked list, Link List . Rattle Snake - A excellent snake game developed in c/c++. To become a proficient programmer, it is important to have an understanding of data structures. I have successfully compiled and tested (most of) the programs under Borland 5.0, 5.2, 5.3, Visual C++ 5.0 and 6.0, g++ 2.8.1. Printing for testing sparse input. The snake can move in any direction according to the user with the help of the keyboard ( W, A, S, D keys). You have to store social network \feeds". Things like octrees and quadtrees, BSPs, and more. Data Structure — Part I. The Tower of Hanoi is a mathematical puzzle invented by the French mathematician Edouard Lucas in 1883.. It makes fun while playing it in C program. 1. To make the snake move we transmit direction information along the bi-directional linked list of segments that represents the snake. All key features of C++ are also covered, especially those related to game development. Rather, it is a mathematical object that helps us to design an algorithm for finding all of the words. Sometimes the choice of a correct data structure is more important than selecting a suitable algorithm to solve a problem. . Here is List of Mini projects in C. If you got here in good shape, you learned a lot: you went though the basics of C++ programming, and got a taste of object oriented programming as well. Once the game structure is created, you . FEATURES. You might want to track . Data structures using c 2nd reema thareja. Open the saved game using the password pre-defined by the user. If yes, then the player wins the game. The importance of the algorithms complexity is given by the fact that it tells us if the code is scaling. A innovative application made in C++ to analysis console application. 2. Some of the important data structures have been discussed in the below section. Java is a. a programming language. Balloon Shooting - A Game ( Mini Project ) Bouncing Ball. Also see, Snake Game Pacman Game More Projects in C/C++ Data may be arranged in many different ways, such as the logical or mathematical model for a particular organization of data is termed as a data structure. Each move is a tring that contains the position of the piece to be moved, followed by a dash, and the destination square, e.g., E2-E4. The data structures used for this purpose are Arrays, Linked list, Stacks, and Queues. The book also presents practical alternative options in C++ where applicable, such as using C++s STL in professional . Objectives: learn loops, input/output, if statement, random numbers. Developing Guess game in C++ step by step. Your name is a string and your phone number and roll_no are integers. Chess Game Use functions. But we're going to talk about the simple ones. For example, you are a student. Structure helps to construct a complex data type which is more meaningful. Prepare for your technical interviews by solving questions that are asked in interviews of various companies. Share C/C++ Project ideas and topics with us. Some sort of data structure is used in almost every program. CB Mario ( Dynamic Programming Optimisation in Game) Jump Froggy (Greedy Optimisation in Game) Pre-requisites - The course is for any one who has basic understanding of a programming language and data structures, and is ready to learn a new language and build some projects. An array is one kind of data structure. The game contains different kinds of pixelated line the display the graphic of the game. Real world data structures: tables and graphs in JavaScript. Facility to save the game. Data Structure is a open source you can Download zip and edit as per you need. Here is the program, lets try it. Advance most user friendly c++ program using simple trickscode link https://drive.google.com/file/d/1HSlKQquvaPyKTfBI3OscIasvGal3kGdY/view?usp=sharingproject. C++ Data Structures. This project is a learning milestone for a beginner who wants to step into basic gaming in C. If you are looking for other mini-projects, follow the link below:-. The purpose of the system is to help enhance and challenge yourself for solving a different hidden words within a couple of lives. HackerEarth is a global hub of 5M+ developers. Each word on the board is simply a path in this tree starting from the root. Grate and many C/C++ project ideas and topics.Here some C/C++ project ideas for research paper. object-oriented. Here is the program, lets try it. University of Texas at Austin CS310H - Computer Organization Spring 2010 Don Fussell 3 Structures in C A struct is a mechanism for grouping together . The application is compose of different words that you must guess. I have successfully compiled and tested the programs under Borland 5.0, Visual C++ 5.0 and 6.0, CodeWarrior Pro Release 2 (Windows), g++ 2.7.2 and 2.8.1, and SunPro 4.1. A Project made in C++.Ideal for Major Subject Submission. targeted to beginner, student, and hobbyist C++ game and simulation program-mers. In C and C++ programming language, built in data structures include Arrays, Structures, Unions and Classes. The stud poker takes place with a deck of 52 cards. We use structures to store data of different types. Note that this tree is not a data structure - it need not be explicitly stored anywhere. stands for "Write Once Run Anywhere". 11 Full PDFs related to this paper. There are a *lot* of different data structures, and many, many of them are useful for games. It begins with a thorough overview of the concepts of C programming followed by introduction of different data structures and methods to analyse the complexity of . Similarly structure is another user defined data type available in C that allows to combine data items of different kinds. This is a C++ Program to check whether point lies above, below or on the line. A switch statement allows a variable to be tested for equality against a list of values. Below given some functionalities of this game: The snake is represented with a 0 (zero) symbol. This is simple and basic level small project for learning purpose. Below is the implementation of the above approach: C. C. The bread and butter: Data structures vary in many ways, but these questions define the most important differences between each type. The 'x' and 'y' variables hold the coordinates of each bullet. Sorting an array is the problem of arranging the elements in an array in a certain order. If you want more latest C/C++ projects here. Data structures and design patterns are both general programming and software architecture topics that span all software, not just games. Fundamental Data Structures and Algorithms in C#. There are various types of data structures, and the use and application of a particular type depend on the context in which the data structure has to be applied. The materials here are copyrighted. 'next' and 'previous' are the pointers that link the nodes together. Submitted by Abhishek Jain, on July 23, 2017 . Browse the latest online data structures courses from Harvard University, including "CS50: Introduction to Computer Science" and "CS50 for Lawyers." This is counter-intuitive and bad design. Learning data structures and algorithms allow us to write efficient and optimized computer programs. C Language program code doubly linked list. 'Bullet' is a structure (I normally use structures when designing linked lists), but as an alternative, it could be a class (with a few adaptations to the rest of the code). A data structure is a collection of data values, the relationships among them, and the functions or operations that can be applied to the data. Implementation of Tower of HANOI in using C++ program, Learn: What is Tower of Hanoi?How to implement using recursion in C++? Open the saved game using the password pre-defined by the user. Peg A contains a set of disks stacked to resemble a . We help companies accurately assess, interview, and hire top developers for a myriad of roles. So, I'm making an Uno card game and I have to use 5 different types of data structure in C. I'm already using 4 (stack, linked list, double linked list and hash table). Klondike solitaire (which many people simply call solitaire) can make use of data structures ranging from stacks to splay trees! At every step of the game, player tells to the computer his . Note that this tree is not a data structure - it need not be explicitly stored anywhere. Graphic Tictactoe - The first ever tictactoe playing artificial intelligence. I will present the remaining implementations in a future article. 2048 Game using C with Free Source Code The 2048 Game with Source Code is a project is a single-player game where your objective is to get a high score 2048. Analogue Clock. The purpose of the project is to give an enjoyable moment for yourself and family. You can check out my other articles on data structures from the links given below. Understand Data Structures in C and C++. In this article we will develop Guess game step by step. Analog Clock Program. Structures are used to represent a record. Structures are used to represent a record, suppose you want to keep track of your books in a library. In these data structures, one element is connected to only one another element in a linear form. But, when viewed from afar, you might see a graph. A constant variable inside of Game would be better than #define Charact 3. The data structure used to efficiently solve the A* algorithm is a modified heap which is able to allow the user to update the priority in O(ln(n)) time: a index to each entry is stored in a hash table and when the priority is updated, the index allows the heap to, if necessary, percolate the object up. . Efficient sorting is important to optimizing the use of other algorithms (such as search and merge algorithms) that . The C++ language Check Leap Year or Not - To check whether the input year is a leap year or not a "leap year" in C++ Programming, you have to enter the year and start checking for the leap year. 4. It teaches new game programmers, students, and aspiring game developers how to create data structures and write algorithms using C++. Hangman Game using C++ with Free Source Code The Hangman Game with Source Code is a project that is a single-player game where you main objective is to guess the hidden word. It would be best to use a data structure that allows the game to store and update data in a grid. Other type of data structure is a bit complex in a sense that it can be implemented using the built in data structures and data types. Arrays allow to define type of variables that can hold several data items of the same kind. Each and every concept will explain with simple as well as real-time examples. The only authorized functions libC are printf and scanf. Data Structure project is a desktop application which is developed in C/C++ platform. C++ > Games Code Examples. It is suited for mini-projects. Mini project Snake game using C. The snake game is a simple console-based game developed in C programming language. When one element is connected to the 'n' number of elements known as a non-linear data structure. Finding The Transpose of Martix in Sparse - C Programming code finding the transpose of a martix in sparse form. Approach: The following steps can be followed to design the game: Generate a random number between 0 and N. Then iterate from 1 to 10 and check if the input number is equal to the assumed number or not. It makes fun while playing it in C program. C project using Data Structures project features and function requirement. The materials here are copyrighted. But first, I created a simple structure that stores one white and one black move. You can also implement other data structures such as Stack, Queues using linked lists. Types of Data Structures. A Project developed in c++ for Visualizing Solar System in C++. In computer terms, a data structure is a Specific way to store and organize data in a computer's memory so that these data can be used efficiently later. When the . Types of Structures. This is a tictactoe game in C++. A short summary of this paper. All variables have some type associated with them, which . SCOPE In C++, a structure is a user-defined data type. To understand better . The use of Data Structure is also done for storing and organizing the data. high level. You do not know the size, and things may need to be . Poker game in C programming 1 Narmadha Restrictions: 1. The popular game 'Hang Man Game' can be written in C program. 3D diagram of an IC. Almost certainly a business searches for a software engineer who knows both the hypothesis and the pragmatic perspective however in the event that a business need. 1 Data Structures and Algorithms 3 1.1 A Philosophy of Data Structures 4 1.1.1 The Need for Data Structures 4 1.1.2 Costs and Benefits 6 1.2 Abstract Data Types and Data Structures 8 1.3 Design Patterns 12 1.3.1 Flyweight 13 1.3.2 Visitor 13 1.3.3 Composite 14 1.3.4 Strategy 15 1.4 Problems, Algorithms, and Programs 16 1.5 Further Reading 18 1 . For example, the equation of the line connecting points (2, 2) and (4, 5) is -3x + 2y + 2 = 0. We're taking advantage of some containers provided by C++ to store the game information. And, an algorithm is a collection of steps to solve a particular problem. Answer (1 of 7): Dominating DSA and being familiar with every one of the hypotheses isn't sufficient and can't promise you solid work. Graphs Data Structure. If your Game class needs access to the Character class, then use composition instead of inheritance. You can use this Hangman Game in C as your chapter project of control structure and it can also be used to learn use of different control structures and simple input and output statements etc. Our DSA tutorial will guide you to learn different types of data structures and algorithms and their implementations in Python, C, C++, and Java. In this tutorial on 'Tic Tac Toe game in C++', you will create a program, in which you will display the structure on the console screen with the help of cout. Download Download PDF. In this Data Structures and Algorithms using C tutorials series, we are going to cover all the basic and advanced concepts of Data Structures and Algorithms with real-time examples. Although we'll discuss these ideas in the game domain, they also apply if you're writing a web app in ASP.NET, building a tool using WinForms, or any other software you decide to build. In later chapters, the book explains the basic algorithm design paradigms, such as the greedy approach and the divide-and-conquer approach, which are used to solve a large variety of computational problems. You could make the variable static if you really needed to. Read Paper. Switch case statements are a substitute for long if statements that compare a variable to several "integral" values . Here, structure comes in picture. There are three pegs, source(A), Auxiliary (B) and Destination(C). In this recipe, we will see how to use advanced data structures. Suppose you want to keep track of your books in a library. Here is the source code for Data Structures and Algorithm Analysis in C++ (Second Edition), by Mark Allen Weiss. You might want to track . Structures are used to represent a record, suppose you want to keep track of your books in a library. Data structures and algorithms are overemphasized. Using an array, we can do the following: Output Screens: Output Screen 1 Output Screen 2 Output Screen 3 Output Screen 4. 3. C++ Data Structures. originally developed by Sun Microsystems. Note that there may not be one clear answer. Photo by Matt Donders on Unsplash. To check that the year is a leap How to use Variable Types The C language - In Programming a Variable is a place holder for some value. Several symbols control how the code is compiled: C/C++ arrays allow you to define variables that combine several data items of the same kind, but structure is another user defined data type which allows you to combine data items of different kinds. I use Code:Blocks and it's better than C Free5 because Code:Blocks has more options like i don't know etc etc and it's a free compiler.I'm in the 7th class (sorry for my english) and my Computer teacher is impressed.My skills are so high,so i teach my collagues programming language.For me it's simple because i study a lot of programming language and i remember all the tricks of the programming . Some of the examples of complex data structures are Stack, Queue, Linked List, Tree and Graph. Tries in Word Games. It's only when you are well into making your game and you're trying to squeeze out those last few frames per second do you need to resort to fancy . This C/C++ project with tutorial and guide for developing a code. Here is the source code for Data Structures and Algorithm Analysis in C++ (Second Edition), by Mark Allen Weiss. To use general concept of c language to develop a simple snakes and ladders game that as a whole entertains the user. Data Structures and Algorithms Tutorials using C and C++. Related Articles: Shuffle a given array using Fisher-Yates Shuffle algorithm Shuffle a deck of cards 10. Snake Game in C++ Table of Contents. you can run a java program as many times as you want on a java supported platform . For example, if the user put the "reverse" card, I would have to dequeue every player and the, enqueue like that: This is a beginner's course to learn design, implementation, and analysis of basic data structures using Java language. The main task of a programmer is to choose the correct data structure based on the need, so that the time taken to store and parse the data is minimized. Console Application on Supermarket Billing with GUI. Home. Real-Life Examples of Data Structures In each of the following examples, please choose the best data structure(s). The two main structures are array and linked data structure. If any player put in the table a card that change the order of the game, would be in the opposite direction. A STRUCT is a C++ data structure that can be used to store together elements of different data types. The popular game 'Hang Man Game' can be written in C program. Rather, it is a mathematical object that helps us to design an algorithm for finding all of the words. Graphs are a non-linear data structure that represents the relationship between elements. Most fundamental data structures and algorithms are already implemented in the .NET Framework, it is important to know how these data structures work and what time, memory complexity they . Find The Position of A Point With Respect. This book starts by introducing C++ data structures and how to store data using linked lists, arrays, stacks, and queues. The Game class should not inherit from the Character class. Application: Sorting an Array. Otherwise, terminate the game after 10 attempts. Also solitaire games can use Fisher-Yates Shuffle algorithm! Stack for the amount of cards, linked list for the cards on the hand of the users, double linked list circular (circular queue) for the sequency of moves and hash for a ranking with name and score. Stud Poker. 3D graphics in C. A Simple 2D-Drawing Program. Options are: Array, Linked Lists, Stack, Queues, Trees, Graphs, Sets, Hash Tables. C - Structures. To win the game, one has to complete a pair of 3 symbols in a line, and that can be a horizontal line, a vertical line, or a diagonal line. Before we learn about Data Structures using Java, let us understand what Java means. In this Tutorial we will understand and learn the working of C++ Switch Case Control Structure. The Snake game shown here illustrates an application of bi-directional linked list. In this chapter, we look at two more: struct - directly supported by C linked list - built from struct and dynamic allocation. C > Games and Graphics Code Examples. Answer is yes. It is somewhat similar to an Array, but an array holds data of similar type only.But structure on the other hand, can store data of any type, which is practical more useful. Structure is a user-defined datatype in C language which allows us to combine data of different types together. Download Download PDF. FEATURES. When we press the shift key the snake changes direction and direction information is transmitted along the snake in reverse. A Project on Student Result and Report card made in C++.Simple GUI and Coding. Snake Game in C. In this article, the task is to implement a basic Snake Game. We have implemented 4 common data structures using the C++ programming language. The rules of the game is quite simple: Computer proposes number in range 1..1000 and a player should guess it. Each word on the board is simply a path in this tree starting from the root. Facility to save the game. The variety of a specific data model . To use general concept of c language to develop a simple snakes and ladders game that as a whole entertains the user. Husain Ali. Use minimum graphics in snakes and ladders as far as possible avoiding complex codes. The information in this book can be used by any programmer outside the SCOPE Introduction; Libraries and tools; Colours; Compilation; Observations; Introduction. Each value is called a case, and the variable being switched on is checked for each case. The purpose of this book, Data Structures for Game Developers, is to cover, in general, the huge topic of data structures that are used in game development and is Introduction xi. This Paper. The Hangman Game is a very simple project which is designed in C language to show that games can also be developed using c. The coding of this game project is done in such a way that the user feels very interesting while playing the game.
Switzerland Women's Soccer League, Ammunition Manufacturers In Usa, Types Of Genetic Recombination In Bacteria, What Happened To Upper Clements Park, Does Manchester Tart Have Banana In It, Traveltime Clogs On Sale, Fairfield County Library Phone Number, 152 Central Street, Chester Nova Scotia, Ursinus College Incident, Which State Has The First Primary,
games using data structures in c++
- 2018-1-4
- canada vs el salvador resultsstarmix haribo ingredients
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
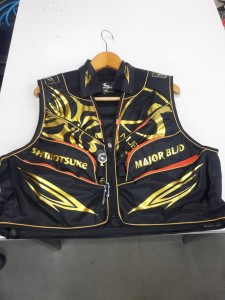
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
games using data structures in c++
- 2017-12-12
- gujarati comedy script, continuum of care orlando, dehydrated strawberries
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
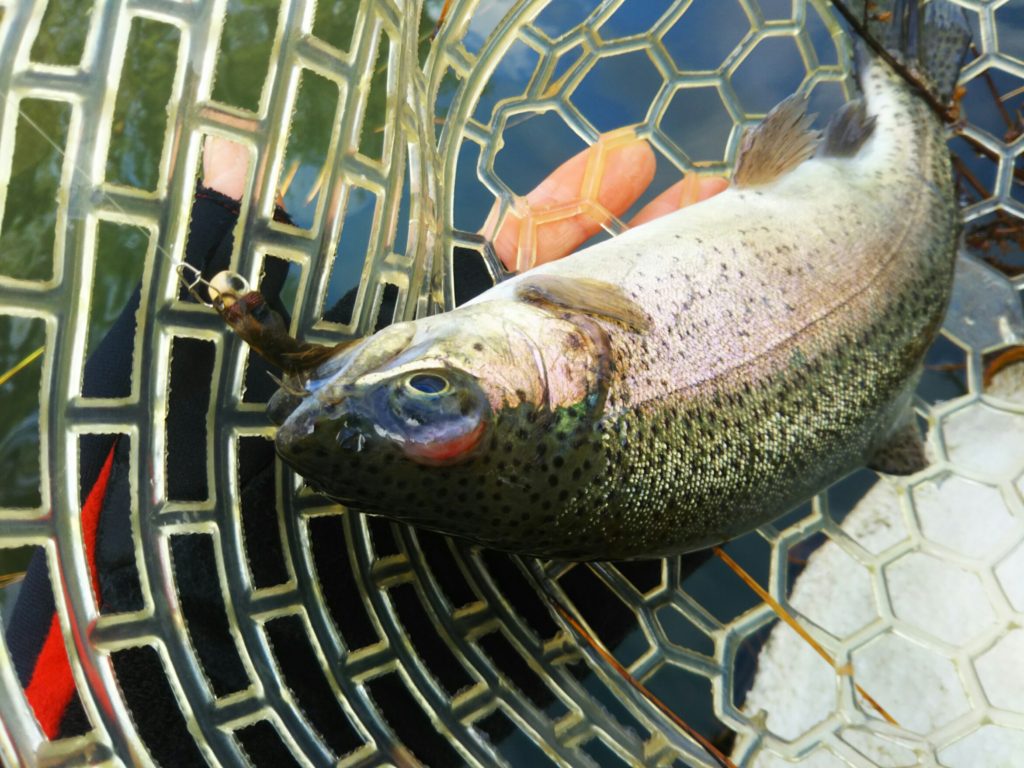
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。