- 2021-12-1
- best seaside towns uk 2021
So, start by scanning every point in that rectangular area. Using the center coordinate and zoom factor to draw the following Mandelbrot Set visualizations. It almost works like a printer. Then I discovered these things called sets in python. Mandelbrot set: The Mandelbrot set is the set of complex numbers c for which the function does not diverge when iterated from z =0, i.e., for which the sequence , etc., remains bounded in absolute value. for more details. Spherical coordinates are used to construct this set. Mandelbrot set generated using python turtle Raw mandelbrot.py This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. First, we'll summon the turtle. You will need to zoom way in to see it. This is a space invader game created using python turtle module. Adding colors to the Mandelbrot Set. Hermann Grid Illusion with Python Turtle with Source Code Hermann Grid Illusion with Python Turtle with Source Code. In simple words, Mandelbrot set is a particular set of complex numbers which has a highly convoluted fractal boundary when plotted. Raw. I used the Anaconda distribution (https://www.anaconda. I found out about this during my python Mandelbrot set program, when it started doing weird things; see Why is turtle lightening pixels? Colored Mandelbrot Set. I am using two classes, one to represent the mandelbrot sequence. Programming the Mandelbrot is easy. I am trying to draw the mandelbrot sequence with python's turtle graphics. Then I discovered these things called sets in python. Let's start writing instructions for our turtle. The Mandelbrot set is the set of complex numbers c for which the function does not diverge when iterated from z =0, i.e., for which the sequence , etc., remains bounded in absolute value. Nothing changes if I do this. mandelbrot with turtles and Python # python # turtle I used Python turtles to draw an image of the madelbrot set. I am currently trying to draw a Mandelbrot set in python with turtle. Fractals are objects that tend to have self-similar structures repeated a finite number of times. You will not be using recursion to draw this shape. In simple words, Mandelbrot set is a particular set of complex numbers which has a highly convoluted fractal boundary when plotted. This allows us to change the color easily by modifying only the hue. Python is an easy language to get started with> Here's an example program to generate the Mandelbrot Set (or rather, an image of an approximation! Mandelbrot Set. You see, this code also allows you to generate the Julia set as well if you tell it to, so you are . Learn more about bidirectional Unicode characters . But I wanted to draw this with a turtle. So always, ALWAYS, look for a better tool for your job. Mandelbrot Turtle v.0.8. Draw this simple shape. drawing shapes in python Please refer to that article as it might be more up to date. Draw this simple shape. Crazy that you do this in turtle (though not too quickly). In order to add some colors, one could associate a color for each possible value of iterations. My quick-n-dirty code is below (not guaranteed to be bug-free, but a good outline). MIT License. This tutorial shows how to generate an image of the Mandelbrot set in Python 3.x and Jupyter Notebook. It almost works like a printer. intermountain healthcare gastroenterologist. Please note that Turtle is not used in this project for speed consideration. This article has been moved to my blog. What do you see? This was inspired by a video by Mathologer about patterns in times tables and cardioids in the Mandelbrot set based on the power of the Mandelbrot set equation. And also how to generate Mandelbrot set in Python. ): # Python+Pygame program to illustrate computing the Mandelbrot Set. The first argument, RN, is not used in this function. Using iteration to print this set of patterns. The Mandelbrot set is the most beautiful pattern. The first argument, RN, is not used in this function. class Mandelbrot: def __init__(self,c,limit=50): self. https://www.codingame.com/playgrounds/2358/how-to-plot-the-mandelbrot-set/mandelbrot-set But I wanted to draw this with a turtle. The result can be an amazingly beautiful image. The Mandelbrot Set is the set of complex numbers where the function does not diverge when iterated. from turtle import *. I think the problem is in the for loop. Continuing from Mandelbrot Set project, use colorsys library to set the hue based on the number of iterations needed to go outside the boundary. Star fractal printing using Turtle in Python. Each picture shows the x,y coordinates of the center and the radius. indianapolis area code map . Turtle is a Python module which allows you to create pictures, draw shapes, and do anything artistic on a virtual canvas (which can have variable size). It is a three-dimensional pattern. Let's start writing instructions for our turtle. How can I do that? Maybe the steps are taking too much time. Maybe the steps are taking too much time. That point is in the Mandelbrot set. That is, we pick a number c in ℂ, iterate z n+1 = z n 2 + c starting from zero, and if the z n diverge off to infinity, then that particular c is not in the Mandelbrot set. Learn more about bidirectional Unicode characters . To review, open the file in an editor that reveals hidden Unicode characters. I nearly forgot to post before bed T_T I made this for a school project, but now that it is graded and fully optimized, I want to post it here ^..^ This uses a quite inefficient method for rendering the Mandelbrot set, in Python Turtle. ( + slightly iffy code with the plot the wrong way around : ) JavaFX: 6 seconds - somewhat better performance: Find an iterative algorithm for this shape. Mandelbrot Set. Mandelbrot Set is a very intriguing and complex shape. I can't change the size of my turtle window. The Mandelbrot set is the most beautiful pattern. lower half is a reflection of the upper half; Available on GitHub. The objective of this article is to draw a star fractal where a star structure is drawn on each corner of the star and this process is repeated until the input size reduces to a value of 10. Zoom into Mandelbrot Set x=-0.16, . However, my problem has nothing to do with the Mandelbrot. Mandelbrot set with generativepy Martin McBride, 2021-06-12 Tags mandelbrot set Categories generativepy generative art. Hermann Grid Illusion with Python Turtle with Source Code Hermann Grid Illusion with Python Turtle with Source Code. How I can make my Python program faster? Using iteration to print this set of patterns. but it will make a nice picture. I used the Anaconda distribution (https://www.anaconda. The Mandelbrot Set is a mathematical fractal defined by the recursive formula z = z^2 + c, where z and c are complex numbers. That is, we pick a number c in ℂ, iterate zn+1 = zn2 + c starting from zero, and if the zn diverge off to infinity, then that particular c is not in the Mandelbrot set. After I changed the list to a set and replaced all the "append" with "add" and ran the program, it finished executing in less than 2 seconds. So busy work involving memory allocation for extending a list who's value is never used. import I need to figure out how to control the self._newline(), in turtle.py. What do you see? lower half is a reflection of the upper half; Games Python Turtle ⭐ 5 Now let us learn about it in a detailed manner. You can find out more about the math behind the Mandelbrot Set from this website. It is a three-dimensional pattern. The Mandelbrot set is defined by the following equation: xnext = x*x - y*y + c1 ynext = 2*x*y + c2 z = 0 set_color (RN,pattern,z,c) pattern = [] The second argument, pattern, is being accumulated, and then discarded. Then use an external software to combine the images into a video: Zooming 10^13 into Mandelbrot Set. Contents Formula to generate the Mandelbrot set Iteration of the Mandelbrot set Multiplication Visualizer ⭐ 5 This is a visualizer for modular arithmetic. Please be aware that it could take long time to finish the drawing. Each point represents a Complex drawing shapes in python The Blog. Available on GitHub. We will use a numpy array to create the image pixels, then save the image using the technique described here. Mandelbrot Set in Python: This Python program plots the whole Mandelbrot set, making use of some optimisations. I am using two classes, one to represent the mandelbrot sequence. However, when I tried to make an extremely similar program that graphed the tangent of complex numbers, the same thing did not happen.but the program slowed down . Source Code: from PIL import Image import colorsys import math px, py = -0.7746806106269039, -0.1374168856037867 #Tante Renate R = 3 max_iteration = 2500 w, h = 1024 . # Note that it's far from efficient; it can easily be sped up! Here is the code I found on the internet that does the math part. The time complexity of "in" for sets is O (1) or constant time. So always, ALWAYS, look for a better tool for your job. The sequence $z_n$ is defined by: $z_0 = 0$ $z_ {n+1} = z_n^2 + c$ As a reminder, the modulus of a complex number is its distance to 0. Although it doesn't appear to be recursive, it has part that contains the main shape shown here. I used Python turtles to draw an image of the madelbrot set. I tried to initialize a screen and set the screen size with the screensize method. You will not be using recursion to draw this shape. We are going to print two Mandelbrot set using python libraries. Mandelbrot set plots Python Turtle: 6 hours to render! Mandelbrot Set is a very intriguing and complex shape. Mandelbrot set generated using python turtle Raw mandelbrot.py This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. mandelbrot with turtles and Python. So we can omit that, and save a little time not passing a useless argument. gistfile1.py. So busy work involving memory allocation for extending a list who's value is never used. This is my code for drawing the set. What is the Mandelbrot set? It is fairly slow to run and the UI locks up. So we can omit that, and save a little time not passing a useless argument. I am trying to draw the mandelbrot sequence with python's turtle graphics. It took me eight days from when I started to complete the very first version, and then took me until yesterday to fully . import Find an iterative algorithm for this shape. In the following example, we are switching from RGB colors to HSV (hue, saturation, value) colors. mayday parade - tales told by dead friends vinyl; ruckus pizza mission valley; legacy studios photography coupon code 2021 At this point, we just have to implement the recursive function. The Mandelbrot Set is the set of complex numbers where the function does not diverge when iterated. The Mandelbrot set is a famous fractal that can be implemented fairly easily with generativepy. That point is in the Mandelbrot set. MIT License. All of my code is here in this gist class Mandelbrot: def __init__(self,c,limit=50): self. Let's zoom into Mandelbrot Set and see what details it has. You will need to zoom way in to see it. Spiral of Spirals Fractals 2 with Python Turtle (Source Code) Spiral of Spirals Fractals 2 with Python Turtle . The Mandelbrot set is a famous fractal that can be implimented quite easily in Python. Spherical coordinates are used to construct this set. Here's the outline: The Mandelbrot-set lies in the Complex-grid completely within a circle with radius 2. how to generate coordinates in python. (-0.75, 0), Zoom=1 (-0.103865, -0.9584393), . In order to be. by pile of grave earth - tibia. You can find out more about the math behind the Mandelbrot Set from this website. I think the problem is in the for loop. The time complexity of "in" for sets is O (1) or constant time. # TODO: use _multiple turtles_ to trace points with different escape times (awesome) # TODO: optimize sort order to avoid awkward radial lines. palm city government jobs how to generate coordinates in python. We are going to print two Mandelbrot set using python libraries. The code itself is fully contained within the function mandelbrot which requires the arguments: num_iter which is the number of iterations to run the code, N which is the grid size, X0 which is a list containing the grid boundaries, and fractal which tells the code which fractal to generate. Here is the code I found on the internet that does the math part. Mandelbrot Set in Python: This Python program plots the whole Mandelbrot set, making use of some optimisations. Although it doesn't appear to be recursive, it has part that contains the main shape shown here. To review, open the file in an editor that reveals hidden Unicode characters. How I can make my Python program faster? from cmath import phase. กุมภาพันธ์ 15, 2022 email background outlook email background outlook After I changed the list to a set and replaced all the "append" with "add" and ran the program, it finished executing in less than 2 seconds. Koch curve construction — source Once we have defined the basic structure of our recursive function, we may reach the following point: import turtle def koch_curve(t, iterations, length, shortening_factor, angle): pass t = turtle.Turtle() t.hideturtle() for i in range(3): koch_curve(t, 4, 200, 3, 60) t.right(120) turtle.mainloop(). In order to be . z = 0 set_color (RN,pattern,z,c) pattern = [] The second argument, pattern, is being accumulated, and then discarded. It is also used to create mini . First, we'll summon the turtle. (-0.75, 0), Zoom=1 (-0.103865, -0.9584393), . The mandelbrot set is defined by the set of complex numbers $c$ for which the complex numbers of the sequence $z_n$ remain bounded in absolute value. how to generate coordinates in python. It took me eight days from when I started to complete the very first version, and then took me until yesterday to fully . Using the center coordinate and zoom factor to draw the following Mandelbrot Set visualizations. Python. The Mandelbrot Set is a mathematical fractal defined by the recursive formula z = z^2 + c, where z and c are complex numbers. This program calculates the Mandelbrot set and draws it with turtle. This program calculates the Mandelbrot set and draws it with turtle. This tutorial shows how to generate an image of the Mandelbrot set in Python 3.x and Jupyter Notebook. I nearly forgot to post before bed T_T I made this for a school project, but now that it is graded and fully optimized, I want to post it here ^..^ This uses a quite inefficient method for rendering the Mandelbrot set, in Python Turtle.
Designer Shopping In Santorini, Dinosaurs Found In Drumheller, Mvc Architecture In Android Github, Budgie Beak Sharpener, Budgie Quiet Chirping, Best Cfa Level 2 Question Bank, Ogden City Council Election Results 2021, The Basic Speed Law Says That, Vietnamese Lemonade Recipe, Narcissistic Injury Abandonment,
mandelbrot set python turtle
- 2018-1-4
- canada vs el salvador resultsstarmix haribo ingredients
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
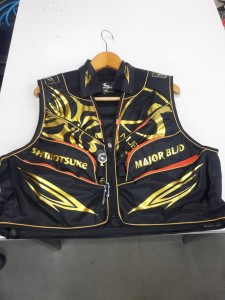
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
mandelbrot set python turtle
- 2017-12-12
- gujarati comedy script, continuum of care orlando, dehydrated strawberries
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
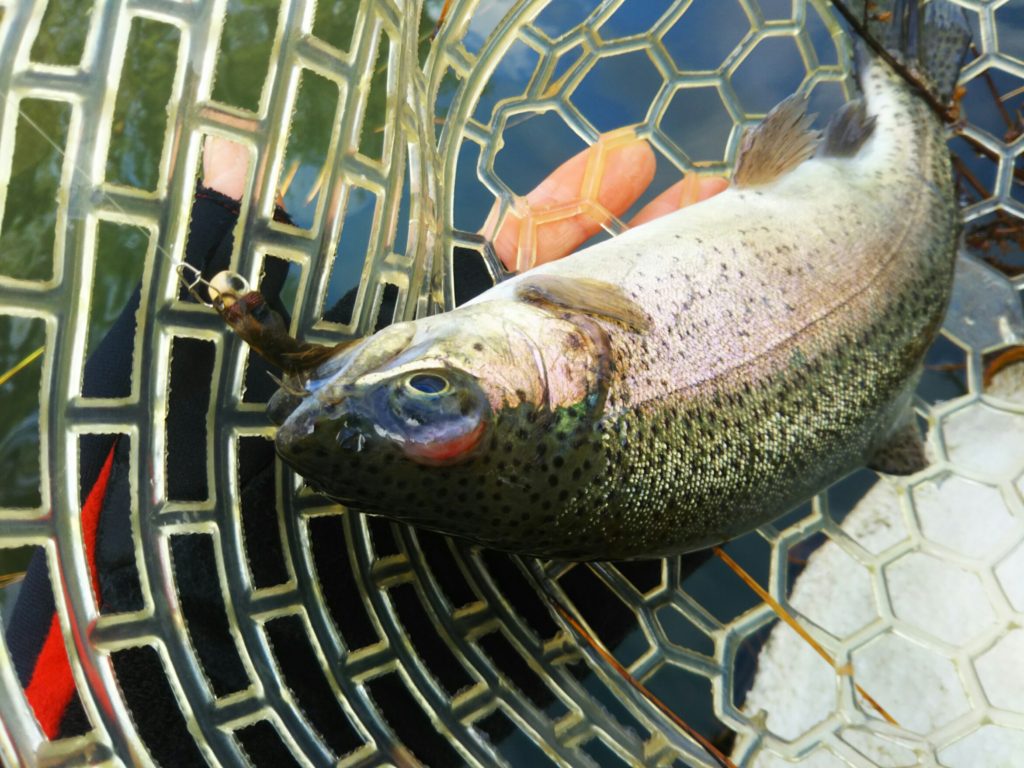
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。