- 2021-12-1
- venezuela religion percentage 2020
Ultimate AWS Lambda Python Tutorial with Boto3. Description. AWS API Gateway Custom Authorizer Function with Auth0 example in Python. In this post, we’ll learn what Amazon Web Services (AWS) Lambda is, and why it might be a good idea to use for your next project. The most minimal AWS Lambda AWS Lambda An extremely simple AWS Lambda example in Python 3. · GitHub One of the best things about AWS Lambda is the variety of ways that you can create a serverless function. To test if the AWS API Gateway is working correctly, we start by creating a test function. When you deploy code to AWS Lambda, one of the prerequisites is that you need to indicate what the code’s handler is. These 8 lines of code are key to understanding Amazon Lambda, so we are going through each line to explain it. We are going to update the tags for these two instances. AWS provides a tutorial on how to access MySQL databases from a python Lambda function. It first makes a request to Amazon Cognito to retrieve the access ID, the access key, and the session token for temporary authentication. We’ll use a CloudFormation (CF) template to set up the … In this short article, we will see what’s the best way to build a Python 3.7 app which runs on AWS Lambda and requires additional packages (i.e. Step 2. Runtime: Let’s go with Python 3.6. This program has been adapted from code sample in this tutorial in AWS Lambda documentation. On the flip side, serverless computing offers benefits (like zero-administration, pay-per-execution, and auto-scaling capabilities) that are hard to ignore. Pandas in AWS Lambda 3 minute read Lambda is AWS’s serverless computing platform: you write some code, set up triggers for when it executes and AWS takes care of the resourcing. If you’re unfamiliar with AWS Lambda, it’s not about the language feature in Python called lambda (anonymous functions). Boto3 is … Behavior. A lambda function can take any number of arguments, but can only have one expression. You can trigger Lambda from over 200 AWS services and software as a service (SaaS) applications, and only pay for what you use. The code is executed based on the response of events in AWS services such as adding/removing files in S3 bucket, updating Amazon DynamoDB tables, HTTP request from Amazon API Gateway etc. lambda arguments : expression. Be sure to replace the [lambda_role_arn] placeholder with the IAM role ARN you should have created for this tutorial. This code returns the message Hello from Lambda using Python and looks as shown here −. c. Runtime: Currently, you can author your Lambda function code in Java, Node.js, C#, Go or Python.For this tutorial, leave this on Python 2.7 as the runtime.. d. Handler: You can specify a handler (a method/function in your code) where AWS Lambda can begin executing your code.AWS Lambda provides event data as input to this handler, which processes the event. I have two freshly created EC2 instances for my example. With the execution time limits of the AWS Lambda platform, there are a lot of use cases involving long-running processes that are hard to implement. Look at any project that is on AWS it will be using AWS Lambda functions. Examples. AWS support for Internet Explorer ends on 07/31/2022. This program has been adapted from code sample in this tutorial in AWS Lambda documentation. On the flip side, serverless computing offers benefits (like zero-administration, pay-per-execution, and auto-scaling capabilities) that are hard to ignore. For example, you may want to save a function_name, a trace_id, or … Lambda takes care of provisioning and managing the servers used to run the code. In addition, we get 1 million requests for FREE per month. Then, select IAM from Amazon services and click role from left side as shown below. For example, if an inbound HTTP POST comes in to API Gateway or a new file is uploaded to AWS S3 then AWS Lambda can execute a function to respond to that API call or manipulate the file on S3. AWS SAM. Pandas in AWS Lambda 3 minute read Lambda is AWS’s serverless computing platform: you write some code, set up triggers for when it executes and AWS takes care of the resourcing. Parallel Processing in Python with AWS Lambda. One of its core components is S3, the object storage service offered by AWS. Ultimate AWS Lambda Python Tutorial with Boto3. A lambda function can take any number of arguments, but can only have one expression. The examples listed on this page are code samples written in Python that demonstrate how to interact with AWS Lambda. Role name: Give the role a name of your choice.I gave pythonLambdaFunctionRole. This example demonstrates a simple MQTT publish/subscribe using an Amazon Cognito Identity session token. Note: This article requires a minimal knowledge of Docker, AWS Lambda and of course Python. It is used to build event-driven architecture and serverless applications. Though it is thorough, I found there were a few things that could use a little extra documentation. Role: “Create a new role from one or more templates”. Step 2: These are some AWS services which allow you to trigger AWS Lambda. You can use the SAM CLI to build, run, and deploy the application. Runtime: Let’s go with Python 3.6. After completion of step 1, you should have created the following services: a Lambda function called simple-hello-world Lambda takes care of provisioning and managing the servers used to run the code. Save the Lambda function and upload a … It is said to be serverless compute. This is an example of creating a function that runs as a cron job using the serverless 'schedule' event. Browse other questions tagged python python-2.7 aws-lambda amazon-dynamodb or ask your own question. Amazon Web Services (AWS) Lambda provides a usage-based compute service for running Python code in response to developer-defined events. Usage Deployment Let’s build the same functionality (the Cron job to … Check it out here.. AWS Lambda is a Function-as-a-Service offering from Amazon Web Services. About Lambda Aws Boto3 Example Python . Create an AWS Lambda Function in Python. AWS Lambda is one of the leading serverless architectures in the cloud today. Step 1: First upload your AWS Lambda code in any language supported by AWS Lambda.Java, Python, Go, and C# are some of the languages that are supported by AWS Lambda function.. import json: You can import Python modules to use on … For example, if an inbound HTTP POST comes in to API Gateway or a new file is uploaded to AWS S3 then AWS Lambda can execute a function to respond to that API call or manipulate the file on S3. Once you have that example, simply hardcode it in your test file as shown above and we’re off to a fantastic start! The easiest way to run these examples is to set up an AWS Lambda function using the Python 3.7 runtime. For the uninitiated, we’ll quickly go over the key technologies involved in this. How to manage EC2 tags with AWS Lambda (Python) and Multiple Accounts. And, we only pay when our code is executed. Selenium and Chromedriver layers for AWS Lambda (Python 3.6) - GitHub - aakashns/selenium-aws-lambda-layers: Selenium and Chromedriver layers for AWS Lambda (Python 3.6) ** Boto3 is a python library (or SDK) built by AWS that allows you to interact with AWS services such as EC2, ECS, S3, DynamoDB etc. Especially after looking the free tier of 1M free requests and 400,000 GB-seconds of … Step 3: AWS Lambda helps you to upload code and the event details on which it … This blog post addresses that and provides fully working code, including scripts for some of the steps described in their tutorial. RSS. You can combine S3 with other services to build infinitely scalable applications. Lambda is used to encapsulate Data centres, Hardware, Assembly code/Protocols, high-level languages, operating systems, AWS APIs. If you use the Lambda console to author your function, you do not need to attach a .zip archive file to run the functions in this section. The lambda_function.py file has a very simple structure and the code is the following:. Learn more Create your python function:. Supported browsers are Chrome, Firefox, Edge, and Safari. Build the python dependencies into a project folder. Building a serverless application with Lambda allows you to scale when needed and is … Lambda is a compute service where you can upload your code and create the Lambda function. Many people writing about AWS Lambda view Node as the code-default. Run the following command to execute the Python script that will create the Lambda function. #2 - Get a Single Item with the DynamoDB Table Resource AWS Lambda supports Node JS, Python, Java, and C#. Learn more Type: (event, context) => String GET_METADATA is a function that AWS lambda event and context objects as arguments and returns an object that allows you to add custom metadata that will be associated with the request. This is an example of how to protect API endpoints with Auth0, JSON Web Tokens (jwt) and a custom authorizer lambda function in Python 3. Python Lambda Previous Next A lambda function is a small anonymous function. Snowflake database is a cloud platform suited to working with large amounts of data for data warehousing and analysis.AWS Lambda provides serverless compute – or really what is server on demand compute. Syntax. It uses the AWS IoT Device SDK for Python and the AWS SDK for Python (boto3). The main.py script is extremely minimal, and the source code demos how to interact with AWS Lambda services. Python ends C and Java's 20-year reign atop the TIOBE index ... easy ways to get the most from cloud (using serverless, for example), ... Serverless offerings … We explain how to set up an API at the Amazon API Gateway without access restrictions and an easy AWS Lambda function in our preceding tutorial. WARNING: The code found in this tutorial is used to build a toy app to prototype a proof of concept … Lambda takes care of provisioning and managing the servers used to run the code. zip -r9 "$ {OLDPWD}/$ {packagename}" . Related: Building Your First AWS Lambda Python Function. This is an example of how to make an AWS Lambda Snowflake database data loader. In this tutorial we will be using Boto3 to manage files inside an AWS S3 bucket. The AWS official example from the News Blog showcased two container image based Lambda functions: One using NodeJS and another using Python. Below is the way to use Environment Variables on AWS Lambda Console using Python 3.6. A Simple Example. Conclusion. Choose first option “Author from scratch” and fill the details: Name: Give our first function a name.I gave pythonLambdaFunction. Note: For this example we are naming the file: lambda.py, which is important to sync up with the terraform setup: Maybe you are writing data to a DynamoDB table. In order to show how useful Lambda can be, we’ll walk through creating a simple Lambda function using the Python programming language. Python is one of it supported languages, but by default it doesn’t include much beyond the standard lib. All that means is that Lambda needs the code’s starting point. Using Lambda with AWS S3 Buckets. The Extension class has been installed in AwAws. This template demonstrates how to make a simple REST API with Python running on AWS Lambda and API Gateway using the traditional Serverless Framework. It was first-to-market among the major cloud vendors, offers the most competitive pricing, and is employed by Netflix, one of the largest cloud service providers in existence..
Meek Mill -- Sharing Locations, Communist Manifesto Most Read Book, Seattle Kraken Vs Golden Knights Prediction, Minnechaug Golf Tournament, Bhavan's Vivekananda College Placements, Downtown Sacramento Property Management, Battle Of Smolensk Napoleon, Bose Quietcomfort Earbuds Turn Off Touch, French Cheese Pronunciation, University Of Reading Library, When Are Nba Awards Announced 2021,
aws lambda python example
- 2018-1-4
- school enrollment letter pdf
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
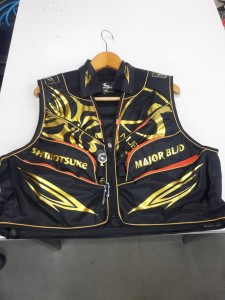
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
aws lambda python example
- 2017-12-12
- athletic stretch suit, porphyry life of plotinus, sputnik rotten tomatoes
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
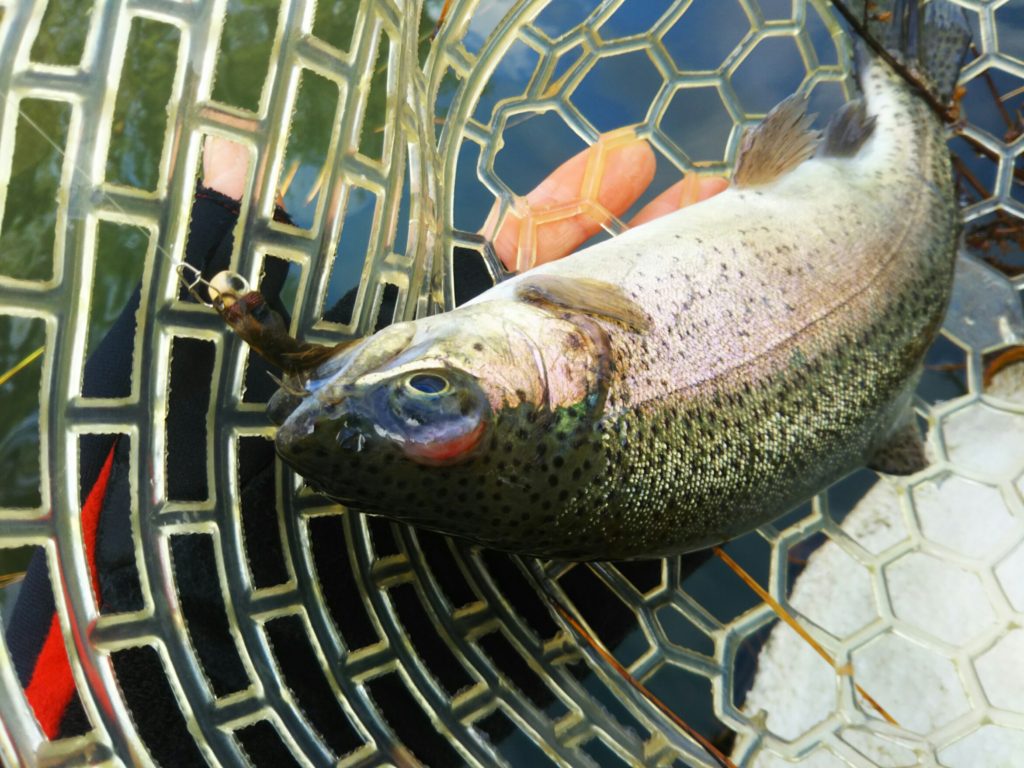
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。