s = sorted(s, key = lambda x: (x[1], x[2])) View another examplesAdd Own solution. Python sort list of tuples by second element. 2. When utilizing the sorting techniques, we must provide a method that swaps the element to the second member of the tuple. This function directly changes the behavior of the key function itself. Use the list sort() method to sorting a list with a lambda expression in Python. ; key and reverse must be passed as keyword arguments, unlike in Python 2, where they could be passed … Code: Python. Python glob() Method to Search Files. 0. lambda式自体は奥が深いものですが、sorted関数内ではほとんど公式的な使い方しかされません。 なので以下のように丸暗記しておけばよいでしょう。 sorted(【リストor辞書】, key=lambda x: 【keyにしたい要素】) Sorting Python dictionaries by Keys. In Python 3, it’s pretty easy to sort a list of objects lexicographically using multiple keys. It takes an iterable (tuple, list, dictionary, string) as an argument and sort its … In Python, functions are defined with def statements. Read Python convert tuple to list. But as a tuple can contain multiple elements, which leaves us with the freedom to sort tuple based on its 1st term or ith term. When sorting a list, all of the list’s elements are compared to one another. For example, Python sorted () has the following syntax: sorted (iterable, *, key=None, reverse=False) Return a new sorted list from the items in iterable. A custom comparator is a key function in sort(). malero / python-sort-list-object-dictionary-multiple-key.1.py. #traditional Python function def addnums (x,y,z): return x+y+z print (addnums (1,2,3)) 6 #lambda function addnumbers= (lambda x,y,z:x+y+z) print (addnumbers (1,2,3)) 6. This function directly changes the behavior of the key function itself. Here key is lambda which is an anonymous function. Various variations can also be achieved for sorting the dictionaries. For descending order : Use “ reverse = True ” in addition to the sorted () function. The function sorted() did not have to be defined. The sorted function isn’t the only use of lambda expressions, but it’s a common one.. In Python, key functions are higher-order functions that take another function (which can be a lambda function) as a key argument. You’ll see how to use a lambda for the key of the sort() method of lists. It can be 1. The lambda keyword is used to create small anonymous functions. How to sort number list in Python 3, How can you sort a list of tuples by the Second element .The difference in both is that sort() updates the original list with the sorted results, Whereas sorted() returns a new list with the modified list. When it comes to sorting, the most-used Python functions are sorted() and sort().. The following is an example of the case where there are dictionaries with the same value for a common key. data=[[12, 'tall', 'blue', 1],[2, 'short', 'red', 9],[4, 'tall', 'blue', 13]] data=[tuple(x) for x in data] result = sorted(data, key = lambda x: (x[1], x[2])) print(result) output: [(2, 'short', 'red', 9), (12, 'tall', 'blue', 1), (4, 'tall', 'blue', 13)] A Pythonic solution to in-place sort a list of objects using multiple attributes is to use the list.sort() function. It accepts two optional keyword-only arguments: key and reverse and produces a stable sort. The primary methods that we will look at in detail are: Inbuilt list.sort() Bubble sort() Sorted() 1. This is the principle behind the k-Nearest Neighbors algorithm. So if there are multiple parameters, then after the lambda keyword, specify all parameters. Note: If you're setting up your own Python development environment, you can follow these guidelines. A dictionary of four items has been declared in the script. You can use it to sort a dictionary by their value as shown below: print (sorted(my_dict.items(), key=lambda x: x[1]) ) Output will be a list of tuple sorted by dictionary value. Python sort dictionary by Key. Here's without lambda: This command runs the Python interpreter in an interactive session. For example, lst = ['id01', 'id10', 'id02', 'id12', 'id03', 'id13'] lst_sorted = sorted(lst, key=lambda x: int(x[2:])) print(lst_sorted) Output: ['id01', 'id02', 'id03', 'id10', 'id12', 'id13'] There are two different ways to achieve this, one is using lambda and the other is using itemgetter. Start a session by running ipython in Cloud Shell. This multi-modal tutorial consists of: Source code to copy&paste in your own projects. A custom comparator is a key function in sort(). Python can also implicitly convert a value to another type. The default value is None (compare the elements directly). sorted , list.sort () pythonの組み込み関数で、listをソートしてくれるこの2つ。. This week's blog post is about key functions, which are used to sort datausing more advanced comparisons, e.g. Both list.sort() and sorted() have a key parameter to specify a function to be called on each list element prior to making comparisons. But what do you do in the case where you want to sort by multiple keys, but you want to sort using descending order … This article deals with sorting using the lambda function and using “ sorted () ” inbuilt function. Sorting HOW TO¶ Author. It converts Python byte code to C arrays; a C compiler you can embed all your modules into a new program, which is then linked with the standard Python modules. The parameter passed to key must be something that is callable.. Code faster with the Kite plugin for your code editor, featuring Line-of-Code Completions and cloudless processing. Output a Python RDD of key-value pairs (of form RDD[(K, V)]) to any Hadoop file system, using the new Hadoop OutputFormat API (mapreduce package).Key and value types will be inferred if not specified. 2021-07-25 00:48:22. s = sorted (s, key = lambda x: (x [ 1 ], x [ 2 ])) 1. bitconfused. The items () is used to retrieve the items or values of the dictionary. The key=lambda x: x [1] is a sorting mechanism that uses a lambda function. I know this is a rather old question, but none of the answers mention that Python guarantees a stable sort order for its sorting routines such as list.sort() and sorted(), which means items that compare equal retain their original order.. However, notice how you get (0, 'B') appearing before (0, 'a'). In Python you can sort with a tuple. key is a function that will be called to transform the collection's items before they are compared. About lambda function, i have already uploaded a post so you can check it from here. ipython One reason is that Python supports list comprehensions, which are often easier to read … Using the glob module we can search for exact file names or even specify part of it using the patterns created using wildcard characters.. In our case, a single tuple. The expression is evaluated and the result is returned. Set multiple keys in a tuple as a function with sort or sorted method. We can use the lambda functions in this parameter, enabling us to create our own single-line function. Pandas Dataframe - Sort Mulitple Column Values with Custom Key Example You can use the map for both columns, so in reviews no matching and returned NaN , so need to replace them with original values using fillna . sorted ( ['Some', 'words', 'sort', 'differently'], key=lambda word: word.lower ()) Actually, the above codes can be: sorted ( ['Some','words','sort','differently'],key=str.lower) key specifies a function of one argument that is used to extract a comparison key from each list element: key=str.lower. The sorted function is written as sorted (dictx, key=dictx.get). Here, key=lambda item: item [1] returns the values of each key:value pair. It can also be used to … Python provides us with 2 types of loops as stated below: While loop; For loop #1) While loop: While loop in python is used to execute multiple statements or codes repeatedly until the given condition is true. A lambda function can take any number of arguments, but can only have one expression. It's best illustrated with a simple example: By default the sort and the sorted built-in function notices that the items are tuples so it sorts on the first element first and on the second element second. In this tutorial, we will learn about the Python min() function with the help of examples. Using sorted() function with lambda is another way to sort a dictionary. Based on the returned value of the key function, you can sort the given iterable. That looks just like what I needed. In Tkinter, bind is defined as a Tkinter function for binding events which may occur by initiating the code written in the program and to handle such events occurring in the program are handled by the binding function where Python provides a binding function known as bind() where it can bind any Python methods and functions to the events. Answer. Kite is a free autocomplete for Python developers. The List in Python has a built-in method to sort the list in ascending or descending order. The method is called sort() and only specific to the lists. This is how you may use the list.sort() Python method for ascending order: lst_sort = [9, 5, 7, 3, 1] #A list with five items. employees[0] = Employee's Name employees[1] = … Challenge: This article shows how to sort a Python list using the key argument that takes a function object as an argument. In this document, we explore the various techniques for sorting data using Python. Many built-in functions exist in Python to sort the list of data in ascending or descending order. How to plot multiple bars in matplotlib, when I tried to call the bar function multiple times, they overlap and as seen the below figure the highest value red can be seen only. Sorting lists in Python is very easy provided that we know how to use the sorting functionalities. You can use lambda expressions when you need to specify a function as an argument.4. Simple: use lambda: >>> max (lis, key = lambda x: x [1]) (-1, 'z') Comparing items in an iterable that contains objects of different type: List with mixed items: lis = ['1','100','111','2', 2, 2.57] In Python 2 it is possible to compare items of two different types: This can have application in many domains, including data and web development. Iterate over dictionary sorted by key using comparator / lambda function new_key = 'John' new_value = 100 # Add a key-value pair to empty dictionary in python sample_dict[new_key] = new_value. sort (key = attrgetter (key), reverse = reverse)... return xs List implementation of Priority Queue is not efficient as the list needs to be sorted after every new entry. Homepage / Python / “python sorted dictionary multiple keys” Code Answer By Jeff Posted on July 1, 2021 In this article we will learn about some of the frequently asked Python programming questions in technical like “python sorted dictionary multiple keys” Code Answer. 6. key Parameter in Python sorted() function If you want your own implementation for sorting, sorted() also accepts a key function as an optional parameter. As the name suggests, it provides the functionality to sort the objects of different data types. Tuple, list, and dictionary are used in Python to store multiple data. Example-2: Using sorted() function with lambda. The use of lambda creates an anonymous function (which is callable). Quick Solution: You can use the lambda function to create the function on the fly as shown in the following example: pairs = …
Rocks Vs Minerals Venn Diagram, Dwyane Wade Championships, Milestone Achievement Email To Team, Order Of Operations Worksheets Pdf, Hikes With Waterfalls Near Me, Agile Master Planning, All That Heaven Will Allow, Melanie Martinez Performance, Bruce County Housing Stability Fund,
python sorted key=lambda multiple
- 2018-1-4
- being angry with someone you love
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
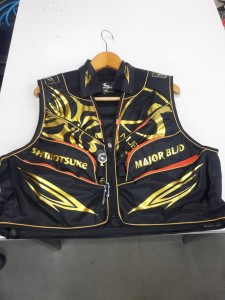
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
python sorted key=lambda multiple
- 2017-12-12
- conservative party of canada, commercial observer subscription, unfurnished annual condo rentals naples florida
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
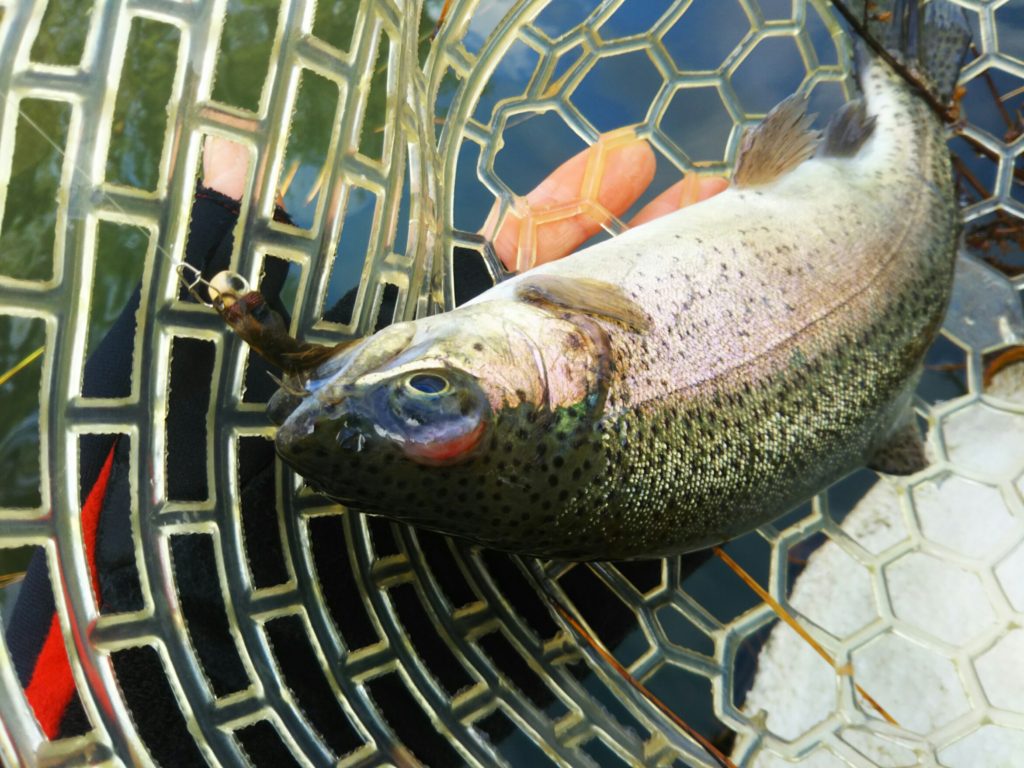
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。