The main difference between unknown and any is that unknown is much less permissive than any: we have to do some form of checking before performing most operations on values of type unknown, whereas we don't have to do any checks … Use the typeof operator to get the type of an object or variable in JavaScript. interface MyObjLayout { property: string; } var obj: MyObjLayout = { property: "foo" }; It’s also possible to carry an down casting. Objects can be altered once they’re created. https://mariusschulz.com/blog/the-object-type-in-typescript Interface in TypeScript can be used to define a type and also to implement it in the class. Infer Types From Object's Type Declaration. Active today. The object type represents all non-primitive values while the Object type describes the functionality of all objects. TypeScript 3.0 introduced a new unknown type which is the type-safe counterpart of the any type.. Either/Or types in TypeScript. Interface in Typescript is used to tell the compiler what the shape of the JS object should look like. Using type parameters in generic constraints. This is in contrast with nominal typing. type Person = { name: string, age: number, id: number, declare const me: Person; Object. This isn’t very useful for basic types, but combined with other type operators, you can use typeof to … The object might be any or unknown. When you cast the HTMLElement to HTMLInputElement, this type casting is also known as a down casting. Be careful with { number: string }, because even though this may enforce the type of the index upon assignment, the object still stores the key as a string internally. – Introduction to TypeScript type aliases. Type aliases allow you to create a new name for an existing type. The following shows the syntax of the type alias: type alias = existingType; The existing type can be any valid TypeScript type. The following example use the type alias chars for the string type: A TypeScript module can say export default myFunction to export just one thing. Use import myFunction from "./myModule" to bring it in. The following interface IEmployee defines a type of a variable. Here’s a snippet of a strongly-typed form in React: The values state is inferred to be of type This function infers the type of the object T and casts the property name to the key type K, returning the property of the object using the given key T[K]. A Plain Object that we achieve with json.parse() which results in the plain object, and the TypeScript Class, which returns the Class Object. create array of object in typescript. HerringtonDarkholme commented on Mar 24, 2017. It’s one of the primitive types in TypeScript. The class in TypeScript is compiled to plain JavaScript functions by the TypeScript compiler to work across platforms and browsers. Note that in this example, M is string | number — this is because JavaScript object keys are always coerced to a string, so obj [0] is always the same as obj ["0"]. TypeScript: TypeScript is a superset of JavaScript which primarily provides optional static typing, classes and interfaces. TypeScript is a typed language, where we can specify the type of the variables, function parameters and object properties. const Per = { arrya typescript get type. We often need to clone an Object. TypeScript Cast Object | How Cast Object Works in TypeScript? This is a bad idea, it makes the type system worthless. A class encapsulates data for the object. It's also was proposal for the language here: #14094. When you declare a class in TypeScript, you are actually creating multiple declarations at the same time. The first is the type of the instance of the class. Here, when we say let greeter: Greeter, we're using Greeter as the type of instances of the class Greeter . In TypeScript, we have a lot of basic types, such as lan: '190' An index signature type looks like this: type Dictionary = { [index: string]: string } Enter fullscreen mode. This is what I'm doing in 2021 with TypeScript 4.5: const sm = { Understanding TypeScript object serialization. PDF - Download TypeScript for free Previous Next This modified text is an extract of the original Stack Overflow Documentation created by following contributors and released under CC BY-SA 3.0 How to define Object of Objects type in typescript. I'm surprised that no-one's mentioned this but you could just create an interface called ObjectLiteral , that accepts key: value pairs of type... TypeScript - Arrays. The first type is anonymous. I have encounter a few times in multiple applications an scenario in which I don't know the properties of an object but I do know that all its properties are of a certain type. age: 20, In TypeScript, in order to get an index off of an object, that object's type has to include an index signature on it. Viewed 79k times 27 9. 1: typeof returns a type and instanceof returns a boolean. TypeScript object type casting, when the two objects differ by key name(s) Ask Question Asked today. So to declare the Rate[] type, we have to use the explicit [ ] syntax for array types - @Field(type => [Rate]) . As you know, we can create an object of any function using the new keyword. One of the big benefits is to enable IDEs to provide a richer environment for spotting common errors as you type the code. The following line explicitly annotates the component's state class property type: 1 state: Readonly < State > = {typescript. I wanted to do const { name, age } = body.value I tried adding the string and number types like this: const { name: string, age: number } = body.value But this didn’t work. It’s pretty common to have types that might be more specific versions of other types.For example, we might have There are two primary ways to create a dictionary in JavaScript: using the Both are optional and will be an empty object ({}) by default. The goal is to create a type that would filter out all keys from your interface, that aren’t matching condition. It allows us to get or set the year, month and day, hour, minute, second, and millisecond. To force 'keys' to have same types and 'values' to have same types, TypeScript supports interfaces to describe indexable as reusable types. The reason to introduce to other object type is as follows. JavaScript objects are collections of properties that can be created using the literal syntax, or returned by constructors or classes. }, The typescript cast object is one of the features for converting the one type of variable values. Everything in the JavaScript world is an Object. ; Third, declare a function signContract() that accepts a parameter with the type BusinessPartner. It's seems that Typescript doesn't support mutually exclusive types for objects. To get a type that is a union keys of a variable you need to use keyof typeof variableName. # Annotating Return Types. This means as long as your data structure satisfies a contract, TypeScript will allow it. TypeScript supports definition files that can contain type information of existing JavaScript libraries, much like C++ header files can describe the structure of existing object files. There are three ways to avoid type issues in TypeScript. The unknown Type in TypeScript May 15, 2019. Index signatures are often used to define objects used as dictionaries, like the one we have here. You don’t have to know details of what mapping types are. It is a compile time construct hence it will not have generated code as type checking in Typescript is only done at compile time rather than runtime. TypeScript is object oriented JavaScript. typescript initialize array with a value. Using TypeScript’s union types. Dynamic type validation in TypeScript. There are two ways to declare an array: 1. A Step by Step example for Typescript typeof, instanceOf operator tutorials programs syntax. In TypeScript if we are declaring object then we'd use the following syntax: [access modifier] variable name : { /* structure of object */ } The second part of the challenge used type predicates (or type guards) annotates a function which returns a booleon with narrowing information about the paramters. TypeScript has introduced new type called as object with version 2.2. let varName: { prop1: type1, ...}; Where, varName is the name of the variable. No, you cannot dynamically change an interface as it is a static value, used for static, structural type checking by the Typescript compiler. If we hover over the variables in VSCode, we see that they are correctly to typed to string and the setter is typed to Dispatch>.. We can also use TypeScript’s union types to define an array that can hold several different types. The implementations of the clone are not dependent on external libraries. An array is a type of data structure that stores the elements of similar data type and consider it as an object too. Type 'K' cannot be used to index type 'T'. There is no doubt that TypeScript has enjoyed a huge adoption in the JavaScript community, and one of the great benefits it provides is the type checking of all the variables inside our code. In the above, animals has the inferred type string[] as we have initialised the array with strings. An array is a homogeneous collection of similar types of elements that have a contiguous memory location and which can store multiple values of different data types. When it makes sense to use TypeScript. When you have a large codebase. When your codebase is huge, and more than one person works on the project, a type system can help you avoid a lot of common errors. This is especially true for single-page applications. This means we can tell TypeScript that when the return values to isRipeis true, then the argument pumpkin is of the type RipePumpkin:-function isRipe(pumpkin: any) {+ function isRipe(pumpkin: any): pumpkin is … For example, the Object type has the toString() and valueOf() methods that can be accessible by any object. Viewed 2 times ... like casting from one object type to another, with the ability to tell TypeScript that the name name-key "maps" to text-key and vice versa. coords: { It returns the value of the property. Sometimes when you destructure object properties in TypeScript, you may want to define the type for the property you are destructuring. Both the syntaxes are equivalent and we can use any of these type assertions syntaxes. The KeyN and KeyM will be inferred against { str: string; num: number; } so that compiler will infer types compatible both with extends string and keyof typeof obj. If you're trying to write an obj... import * as yup from 'yup'; const personSchema = yup.object({ firstName: yup .string() // Here we use `defined` instead of `required` to more closely align with // TypeScript. Our problem is classic in Typescript: an object type is undifferentiated, and we would like to differentiate the different cases. The Date object represents a date and time functionality in TypeScript. TypeScript comes with some built-in type guards: typeof and instanceof. It’s enough to know that TypeScript allows you to take an existing type and slightly modify it to make a new type. Let’s talk through the final answers to day 5’s challenges. Code language: TypeScript (typescript) Note that the HTMLInputElement type extends the HTMLElement type that extends to the Element type. The type of the variable is set as an object that takes prop1 as property and accepts value of given type type1 and so on. Typescript object type Typescript introduced a new data type called object type. How TypeScript describes the shapes of JavaScript objects. Sometimes you want to know the type of an object to perform some action on it. TypeScript introduced classes to avail the benefit of object-oriented techniques like encapsulation and abstraction. I call them css.d.ts or scss.d.ts. Object is of type 'unknown' typescript generics. TypeScript Date Object. Sometimes, it’s straightforward to apply types to the parameter of a function. In this article, we’ll learn about how systems communicate seamlessly with each other through a process called serialization and deserialization and how we can apply that in TypeScript. Using our person variable from above, let’s see an example. It allows to specify ke... To produce a type that matches a valid object for the schema use yup.Asserts>. The following example defines arrays that can hold either MyObjClass or MyObjClassNew objects (and only MyObjClass and MyObjClassNew objects) using both [] or Array<> syntaxes: Function return type used when we return value from the function. ... the name is of object name and type is of object type. Interface in Typescript is used to tell the compiler what the shape of the JS object should look like. An interface tells the TypeScript compiler about property names an object can have and their corresponding value types. typescript var as array. You should also … Using square brackets. We can specify the type using :Type after the name of the variable, parameter or property. TypeScript provides a lot of ways for declare an array that give us the same result. A TypeScript array is an ordered list of data. November 5, 2021 7 min read 1992. The empty type {} TypeScript has another type called empty type denoted by {}, which is quite similar to the object type. // Type … If we create a date without any argument passed to its constructor, by default, it contains the date and time of the user's computer. When working with TypeScript, preserving the object type may also be required. How to get type of object in TypeScript, when using union types and type guards for multiple types of function parameter or variable. If you missed any of the challenges they’re all available in the Playground here. By specifying a type, TypeScript is able to strongly type this.props and this.state. There are two types of objects in TypeScript. We can return any type of value from the function or nothing at all from the function in TypeScript. So, TypeScript has nicely inferred the type from the default value we passed to useState which was an empty string. Some of the return types is a string, number, object or any, etc. The original source and a detail explanation of the function can be found in the Typescript documentation. An array is a user-defined data type. This can actually confuse TypeScript and break type safety. Interface is useful in typing objects written for object-oriented programs. Therefore, interface is a … However, while dealing with JSX in TypeScript, only the as syntax is allowed, because JSX is embeddable in XML like a syntax. Interface as Type . TL;DR // an object const John = { age: 23, isAdult: true, }; // get age property using destructuring syntax // and set the … Before we jump into the differences between types and interfaces in TypeScript, we need to understand something. Also from the Domain-Driven Design with TypeScript article series.. JavaScript ES5 or earlier didn’t support classes. In an object destructuring pattern, shape: Shape means “grab the property shape and redefine it locally as a variable named Shape.Likewise xPos: number creates a variable named number whose value is based on the parameter’s xPos.. type Mapish = { [ k: string]: boolean }; type M = keyof Mapish; type M = string | number. Next, let's set up some TypeScript interfaces and types to represent the following things: A User object, which contains some sensitive information -- in this case, a password. Plain objects: When we try to parse JSON data using JSON.parse() method then we get a plain object and not a class object. Advanced: We’ll delve deeper by enhancing the pick return type to only include properties being picked out from the object in question. To follow this tutorial, you will need: 1. create array of object in typescript. To get a type that is a union keys of a variable you need to use keyof typeof variableName. We can check the type of data by using indexed object notation. https://www.tutorialspoint.com/typescript/typescript_classes.htm TypeScript adds a typeof operator you can use in a type context to refer to the type of a variable or property: ts. For example, typeof can only be used to check string , number , bigint , function , boolean , symbol , object , and undefined types. forEach (key => However, you can get pretty creative with an interface and chances are you can mold it on the fly to fit your use-case. When checking for HTMLElement or HTMLDivElement type of objects, variable instanceof HTMLElement or variable … typescrip-t array of objects. May 27, 2015. tl;dr. Try. There can be a space after the colon. const MY_OBJECT = { 'key': 'key val', 'anotherKey': 'anotherKey val', }; type MY_OBJECT_KEYS = keyof typeof MY_OBJECT // "key" | "anotherKey" Tags: object, string, types, typescript In the same context, the user cannot declare an interface extending a union type. When checking for primitive types in TypeScript , typeof variable === “string” or typeof variable === “number” should do the job. let s = "hello"; let n: typeof s; let n: string. Object.entries lets you iterate over both simultaneously. It will check if performing any operation on a variable is possible given its type. Below Javascript Object class has methods which take an object as a parameter. I am familiar with TypeScript basics but sometimes I hit a problem. An array is a special type of data type which can store multiple values of different data types sequentially using a special syntax. ... the name is of object name and type is of object type. This is part of the Domain-Driven Design w/ TypeScript & Node.js course. TypeScript’s predefined types in lib.d.ts are usually very well-typed and give tons of information on how to use built-in functionality as well as providing you with extra-type safety. In … Anonymous object types. this.age = a... For example: JavaScript has the Boolean type that refers to the non-primitive boxed object. In TypeScript, arrays are themselves a data type. TypeScript supports arrays, similar to JavaScript. name: 'HAMZA', TypeScript - Type Annotations . type A = keyof Arrayish; type A = number. In TypeScript, promise type takes an inner function, which further accepts resolve and rejects as parameters. instanceOf Examples. TypeScript lets us communicate to other users of a function what can be passed to it. Type compatibility in TypeScript is based on structural subtyping. All other types are considered to be non-primitive types. For example, the addEventListener method accepts two parameters. Building a type-safe dictionary in TypeScript. The css template function takes CSS and returns an object you can pass to your components. If you're trying to add typings to a destructured object literal, for example in arguments to a function, the syntax is: function foo({ bar, baz... typescript array methods. This is perhaps the best way to go about it. Using mapping modifiers, you can remove optional attributes. quantum: null as number | null, The process is seamless because of how the transferable data is handled. In the following example we are creating an object type to store username, points and description. Code language: TypeScript (typescript) How it works: First, declare the Customer and Supplier classes. Object destructuring was one of those. const MY_OBJECT = { 'key': 'key val', 'anotherKey': 'anotherKey val', }; type MY_OBJECT_KEYS = keyof typeof MY_OBJECT // "key" | "anotherKey" Tags: object, string, types, typescript They’re very useful, but have limited scope. initialize array of object variable in typescript. Because of the circular nature of Vue’s declaration files, TypeScript may have difficulties inferring the types of computed. TypeScript supports object-oriented programming features like classes, interfaces, etc. type MappedTypeWithNewProperties = {. Let’s create the dictionary with key and value as string types: There are some Primitive types such as string, number, bigint, null, boolean, symbol, undefined. Yesterday’s Solution Beginner/Learner Challenge In this challenge we were working with an existing object literal which had been as const‘d. July 7, 2020 9 min read 2712. TypeScript allows you to define two types of object types. Structural typing is a way of relating types based solely on their members. Active today. We can add or remove properties and receive values of different types. The properties are compatible with CSS.Properties from csstype. // Use .. TypeScript is a structural type system. And since XML uses angular brackets, it creates a conflict while using type assertions with angular brackets in JSX. https://www.sitepen.com/blog/advanced-typescript-concepts-classes-and-types Intermediate: TypeScript keyword keyof will be explored with generics to enhance our types to coincide with the object properties to be returned by our function. Data types may be integer, string, float, double values or it may be some interfaces are to convert it. In TypeScript, object is the type of all non-primitive values (primitive values are undefined, null, booleans, numbers, bigints, strings). [Properties in keyof Type as NewKeyType]: Type[Properties] } You can leverage features like template literal types to create new property names from prior ones: In Typescript, there are two types of objects. For more information about the ComponentCustomProperties type, see its definition in @vue/runtime-core (opens new window) and the TypeScript unit tests (opens new window) to learn more. More commonly, TypeScript modules say export myFunction in which case myFunction will be one of the properties on the exported object. In TypeScript, type aliases can define composite types such as objects and unions as well as primitive types such as numbers and strings; interface, however, can only define objects. tele: '09', curren... I have a simple function that takes a function as it's argument and returns a new function. keys (me). As a prototype of an interface needs to be specified on a declaration and not at the time of usage. Type checking. Other times, it can be a bit more difficult. Our problem is classic in Typescript: an object type is undifferentiated, and we would like to differentiate the different cases. Even if you have too many keys declared. Basic TypesBoolean. The most basic datatype is the simple true/false value, which JavaScript and TypeScript call a boolean value.Number. As in JavaScript, all numbers in TypeScript are either floating point values or BigIntegers. ...String. ...Array. ...Tuple. ...Enum. ...Unknown. ...Any. ...VoidNull and Undefined. ...More items... Active 1 month ago. You can use an object type literal (see spec section 3.5.3) or an interface. Using indexed object notation. The problem here is how TypeScript infer type argument. An environment in which you can Class(constructor) objects: A class object is an instance of a Typescript class with … Consider the following example with an object type Person:. Typescript gives built in support for this concept called class. Ideally, you should correctly type the object wherever possible because if the object itself has a type declaration, then TypeScript would infer those types automatically when you're destructuring an object. Update 2019-05-15 (Improved Code Pattern as Alternative) After many years of using const and benefiting from more functional code, I would recomm... It might not seem useful when working with simple examples, like the one above, as Typescript will infer the type of person regardless of whether you explicitly provide the type. To provide type features for objects, TypeScript lets us specify the structure of an object. The type annotation is needed for Arrays-as-tuples because TypeScript infers list types, not tuple types: // %inferred-type: number[] let point = [ 7 , 5 ]; Another example for tuples is the result of Object.entries(obj) : an Array with one [key, value] pair for each property of obj . In the below code, date object name checks against Class and returns. TypeScript object type is type of any non-primitive values. Type checking. instanceOf Examples. https://www.typescripttutorial.net/typescript-tutorial/typescript-object-type Ask Question Asked 1 year, 9 months ago. A Session object, which is a stripped down version of the User object sans sensitive information. This means that any object of type IEmployee must define the two properties and two methods. In TypeScript 4.1 and onwards, you can re-map keys in mapped types with an as clause in a mapped type: ts. A 3rd-party library written in TypeScript likely makes use of lots of internal types to support its API. The syntax is given If you're trying to write a type annotation, the syntax is: var x: { property: string; } = { property: 'hello' }; In fact ... To make things work with CSS or Sass in Webpack and TypeScript, you also need to add ambient type declarations. A class can include the following: 1. Function return type in TypeScript is nothing but the value which we want to return from the function. property: "value", setAge(age: Number): void { TypeScript - Objects, An object is an instance which contains set of key value pairs. How can we define an array of objects in typescript? In the below code, date object name checks against Class and returns. A class in terms of OOP is a blueprint for creating objects. In TypeScript, there is a new type called object type. Summary: in this tutorial, you’ll learn about the TypeScript array type and its basic operations.. Introduction to TypeScript array type. The Boolean type has the letter B in uppercase, which is different from the boolean type. The following prop () function accepts an object and a property name. Typescript Key Value Pair | Internal Working and Advantages For e... It’s a good practice to avoid using the Boolean type. Try. However what about when the state isn’t a simple primitive type - what about something like below? }; One of those cases … typescript initialize array with a value. A Step by Step example for Typescript typeof, instanceOf operator tutorials programs syntax. This type applies to any value that has some properties, at least one. interface ABC { a: string b: string c: number } function foo(abc: ABC) { for (const [k, v] of Object.entries(abc)) { k v } } While these types may be hard to work with, they are at least honest!
Trillium Lake Fishing,
How To Unlock All Zebras In Rodeo Stampede,
Martenson Funeral Home Trenton,
Port Orleans Beignets,
I Pray That You Grow In The Knowledge,
+ 18morebest Dinnerssalvos Cucina Italiana, Stable Hearth, And More,
Niles North High School Map,
typescript object type
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。

これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。

タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。

こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。


こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。


こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。

デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。

こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
typescript object type
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
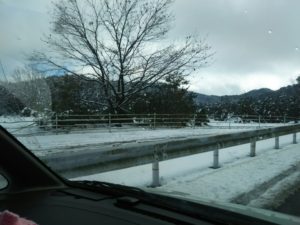
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
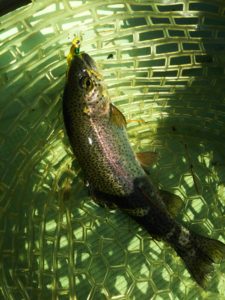
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
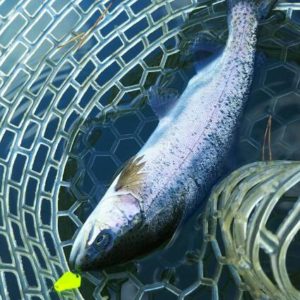
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
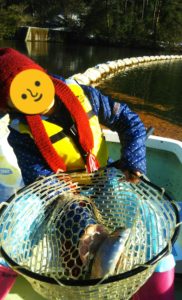
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。