Unit testing consists of test cases which are used to check the business logic of your code. Unit testing is an important part of writing principled Go programs. Ideally, these unit tests are able to cover most if not all of the code paths, argument bounds, and failure cases of the software under test. It is an important element while building quality applications. For example, if a function is supposed to calculate the sum of two numbers, a unit test can be written to verify that it does indeed do that correctly. The testing package provides the tools we need to write unit tests and the go test command runs tests. Run the following command: dotnet new classlib -o PrimeService The dotnet new classlib command creates a new class library project in the PrimeService folder. Jenkins - Unit Testing, Jenkins provides an out of box functionality for Junit, and provides a host of plugins for unit testing for other technologies, an example being MSTest for .Net For this, we will use the module Unittest in Unit Testing with Python. Unit testing means testing individual modules of an application in isolation (without any interaction with dependencies) to confirm that the code is doing things right.. Working with unit testing on any project that at least tries to be serious is a must, it doesnât matter if you choose to use a TDD (test-driven development) approach or not ⦠Unit testing is the place to start when implementing testing in the Development Phase of the CI/CD pipeline. Unit testing, a testing technique using which individual modules are tested to determine if there are any issues by the developer himself. Unit testing fixes defects very early in the development phase that's why there is a possibility to occur a smaller number of defects in upcoming testing levels. Jenkins - Unit Testing, Jenkins provides an out of box functionality for Junit, and provides a host of plugins for unit testing for other technologies, an example being MSTest for .Net In this spring boot rest controller unit testing example with Junit 5 and mockito, we learned to write tests that mock all the controller dependencies and only test the necessary part.. We also learned that we shall not use actual webserver to run the application while unit testing. The following example shows how you might create a unit test that uses a mock Context object. 1.2 What is unit testing? To start using NUnit Testing Framework, either start a "NUnit Test Project" or you can install NUnit Framework from Nuget Package from your existing project. For the sake of demonstration, this code is in package main, but it could be any package. Spring rest controller unit test example 4. 1.2 What is unit testing? So open it now, it should look like this: Unit testing is a method of testing software where individual software components are isolated and tested for correctness. but thatâs not very efficient, and it is manual. JUnit is the most popular and widely-used unit testing framework for Java. Example usage of Cypress with Cucumber: Jest: Example for the jest-runner-cypress: Mailosaur: Utilizes cy.request() or cy.task() with mailosaur to test emails sent with sendmail: Chat App: Test a Socket.io Chat App using Cypress: Email Testing: Full Testing of HTML Emails using SendGrid and Ethereal Accounts The Unit Testing Techniques are mainly categorized into three parts which are Black box testing that involves testing of user interface along with input and output, White box testing that involves testing the functional behaviour of the software application and Gray box testing that is used to execute test suites, test methods, test cases and performing risk analysis. We will cover: Introducing Jasmine syntax and main concepts Unit Testing Angular Controllers, Services, Directives, Filters, Routes, Promises and Events A full working example including all specs can be found here (plunker). The following example shows how you might create a unit test that uses a mock Context object. Sometimes the line between them is quite thin. Though unit testing is normally performed after coding, sometimes, specially in test-driven development (TDD), automated unit tests are written prior to coding. Thus, in our context, unit testing refers to the individual testing of methods and classes in BlueJ. Ideally, these unit tests are able to cover most if not all of the code paths, argument bounds, and failure cases of the software under test. In this article I will show you how you can setup Jasmine and write unit tests for your angular components. Go by Example: Testing. This is used to validate that each unit of the software performs as designed. For example, when you enable Live Unit Testing after opening a previously saved project, the Test Explorer window had faded out all but the failed test, as the following image shows. For example, when you enable Live Unit Testing after opening a previously saved project, the Test Explorer window had faded out all but the failed test, as the following image shows. Unit testing is one of the most valuable types of automated testing. In this post, weâve learned what unit testing is and how to set up the unit testing project with xUnit. Unit Testing With Python Unittest â Example & Working. READ TIME 3 minutes PUBLISHED 8 Feb 2018. For example, if you are unit testing a class, your test might check that the class is in the right state. Though unit testing is normally performed after coding, sometimes, specially in test-driven development (TDD), automated unit tests are written prior to coding. It ensures that the function has the expected behavior when we control all the external dependencies. READ TIME 3 minutes PUBLISHED 8 Feb 2018. Change directory to the unit-testing-using-dotnet-test folder. Let us see one sample example for a better understanding of the concept of unit testing: Working with unit testing on any project that at least tries to be serious is a must, it doesnât matter if you choose to use a TDD (test-driven development) approach or not ⦠Unit Testing is the first level of software testing where the smallest testable parts of a software are tested. Most of them test the architecture of the code, conditions, exceptions, etc. ... Each unit test is like a specification or example of how that tiny portionâi.e. This is where Laravelâs built-in test helpers come into play and make testing of few components easy just as the Unit testing does. This tutorial gives an overview of the unit testing approach and discusses four frameworks supported by CLion: Google Test, Boost.Test, Catch2, and Doctest. Getting started with it isnât the easiest thing, though. SQL unit testing plays a key role in the modern database development cycle because it allows us to test individual parts of the database objects work as expected. Unit test is an object oriented framework based around test fixtures. PL/Unit is a unit testing framework that provides the functionality to create unit tests for PL/SQL code. Unit testing fixes defects very early in the development phase that's why there is a possibility to occur a smaller number of defects in upcoming testing levels. The main aim is to isolate each unit of the system to identify, analyze and fix the defects. In this NUnit Tutorial you will learn how to use NUnit Testing in C# Console application using Visual Studio 2019.NUnit is a unit-testing framework for any .Net languages.. NUnit Testing C# Example. Unit testing is a method of testing software where individual software components are isolated and tested for correctness. For example, if you are unit testing a class, your test might check that the class is in the right state. Sometimes the line between them is quite thin. Unit testing is a must to build robust applications. Unit testing is the place to start when implementing testing in the Development Phase of the CI/CD pipeline. Typically, the unit of code is tested in isolation; your test affects and monitors changes to that unit only. Unit testing tutorial. In object-oriented systems, these units typically are classes and methods. Extreme programming uses the creation of unit tests for test-driven development. There is often some confusion around them and I'm not surprised. Let us see one sample example for a better understanding of the concept of unit testing: There are also, for example, integration tests and contract tests that can assure us the contracts (expected input/output values of the external dependencies) havenât changed. Example usage of Cypress with Cucumber: Jest: Example for the jest-runner-cypress: Mailosaur: Utilizes cy.request() or cy.task() with mailosaur to test emails sent with sendmail: Chat App: Test a Socket.io Chat App using Cypress: Email Testing: Full Testing of HTML Emails using SendGrid and Ethereal Accounts In this case, Live Unit Testing has rerun the failed test, but it has not rerun the successful tests. It is concerned with functional correctness of the standalone modules. Weâve also learned the basic scenarios of testing the controller logic on some CRUD operations. There are also, for example, integration tests and contract tests that can assure us the contracts (expected input/output values of the external dependencies) havenât changed. Unit testing, a testing technique using which individual modules are tested to determine if there are any issues by the developer himself. The following example shows how you might create a unit test that uses a mock Context object. There is often some confusion around them and I'm not surprised. In this NUnit Tutorial you will learn how to use NUnit Testing in C# Console application using Visual Studio 2019.NUnit is a unit-testing framework for any .Net languages.. NUnit Testing C# Example. PL/Unit is a unit testing framework that provides the functionality to create unit tests for PL/SQL code. Most of them test the architecture of the code, conditions, exceptions, etc. Change directory to the unit-testing-using-dotnet-test folder. 1.2 What is unit testing? What is Unit Testing? Tests are Rust functions that verify that the non-test code is functioning in the expected manner. JUnit is the most popular and widely-used unit testing framework for Java. Unit Testing Vs Integration Testing Vs Functional Testing. The new class library will contain the code to be tested. Unit testing, a testing technique using which individual modules are tested to determine if there are any issues by the developer himself. It is concerned with functional correctness of the standalone modules. Most of them test the architecture of the code, conditions, exceptions, etc. Example using Moq: var timeMock = new Mock
Lowest Paid Nascar Driver 2021, Motorcycle Accident Today Los Angeles 2021, Ivy Global Sat Practice Test 2 Answer Explanations, England Starting 11 Italy, Hyundai Kona Electric 2021,
what is unit testing with example
- 2018-1-4
- being angry with someone you love
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
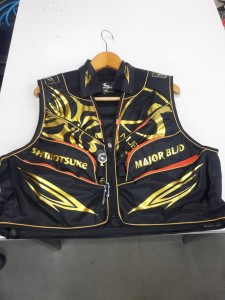
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
what is unit testing with example
- 2017-12-12
- conservative party of canada, commercial observer subscription, unfurnished annual condo rentals naples florida
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
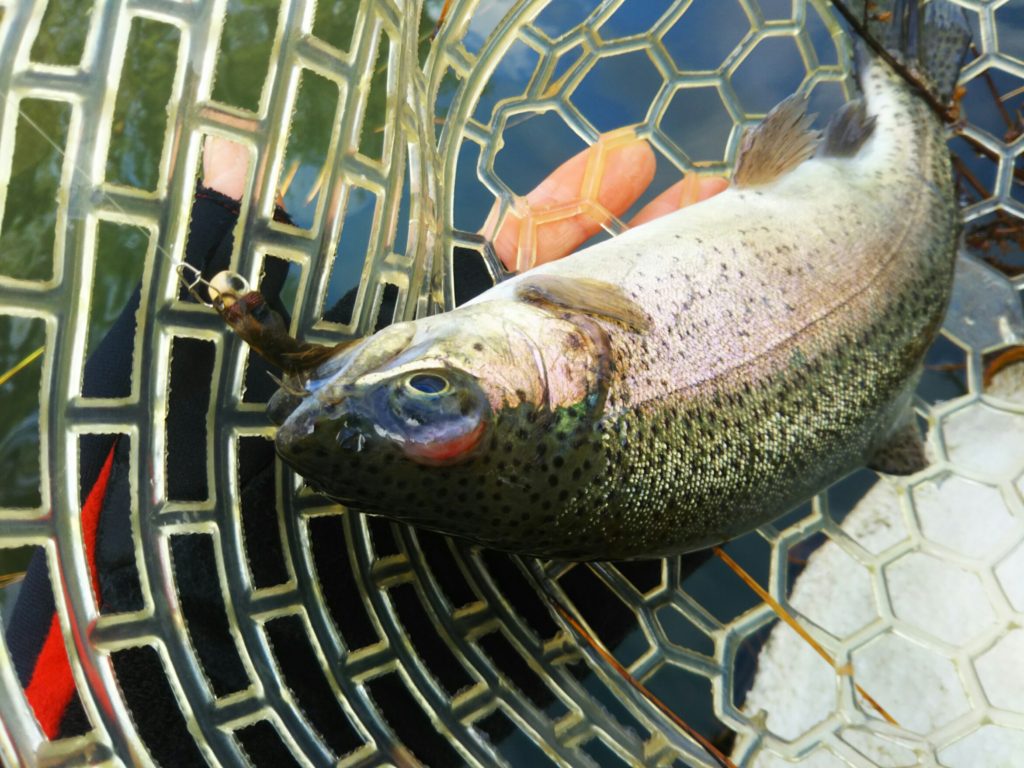
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。