These allow you to structure your views and reuse code by harnessing inheritance and mixins. In this tutorial we will implement basic search in a Django website and touch upon ways to improve it with more advanced options. The View in Django is the business logic layer. It fetches the data from the model, gives each template access to specific data to display, or it could perform some processing over the data beforehand. Django blog tutorial part 2: Model View Template. Django MVT. We will build the home page that lists all the blog posts and a second page that display all the details about a single post. These shortcuts are helper functions that span many different levels of the model, view, template paradigm. mysite > main > admin.py. So, control is handled by the Django framework itself. The T(Template) in the MVT(Model-View-Template) architecture refers to the same concept. admin. Django model form is an efficient way of creating forms using Django models without writing HTML codes. This is in the forms.py file. We are first going to create a django project, an app inside that project by following the below steps:-. in urls.py, we need to add a path of our UpdateView. In this part, we are going to explore the MVT architecture. Templates are responsible for the entire User Interface completely. You can see the video here: YouTube. urls.py. Class-based view. Django Model Crud Operations. Django URLs and Views. success_url: The URL that we'll redirect if the form has been updated successfully. It is responsible for maintaining data. 3. Django has a plethora of so-called shortcut functions that make developing with Django easier. In the second part of this tutorial series, you'll continue to build out a social network with Django. Setup your template to present the data to the user in whatever way you wish. Django (named after the Django Reinhardt) is a high-level python-based free and open-source web framework that follows the model-view-template(MVT) architectural pattern. On Standard ways the templates used in a python setup are rendered using a Django view. If you have not done so already, map a URL to your view. MVT is a software design pattern for developing web applications. You can also render out templates. Django follows MVC pattern very closely but it uses slightly different terminology. 23 Comments. urls.py. Gain knowledge about the Django web app . in this example, we'll display detail of records dynamically. I try to make the least amount of assumptions possible while still trying provide a useful setup. models.py. Before improving our template, we must send variables to the templates. This is a simple Django 3.0+ project template with my preferred setup. 1. The template uses the for and endfor template tags to loop through the book list, . Here as already mentioned, Django works as a controller and gets a URL that is linked to the view part of the application and thus transports the user responses to the application. The render () function is one such function. In the first section of this chapter, you can see in figure 10-5 a selection grid to assign add, change and delete permissions on individual models to each user in a Django project. It follows the Model-View-Template(MVT) design pattern. Download the file for your platform. Just like View in MVC, Django uses templates in its framework. To use it, we must import admin: from django.contrib import admin. Step 1 : We will change our view to inject variables in our template. Django tries to take away some of that monotony at the model and template layers, but web developers also experience this boredom at the view level. Python-Django (views, templates & models) Django is a rich web framework written in python. Aug 6, 2019. There's no difference between MTV and MVT—they're two different ways to describe the same thing, which adds to the confusion. Django Search Tutorial. It is the logical data structure behind the entire application and is . Download files. django admin startproject blogdownloadfiles. CRUD in general means Creating, Retrieving, Updating and Deleting operations on a table in a database. Django is mainly an MTV (Model-Template-View) framework. Create a Django project and an app, I named the project "multipleFormHandle" and the app as "formhandlingapp". The . model: The model that you want to modify. . Define a class-based view BookCreateView and inherit from built-in generic view BSModalCreateView.BookCreateView processes the form defined in #1, uses the template defined in #2 and redirects to success_url showing success_message.. views. template_name: The template's name in that you'll create the records update form. Create Django Project. Django uses the term Templates for Views and Views for Controller. The template is a HTML file mixed with Django Template Language (DTL). Instagram, one of the most prolific social media application use the Django framework. Note: I gave a version of this tutorial at DjangoCon US 2019. Django provides a test framework with a small hierarchy of classes that build on the Python standard unittest library. Model manages database users and admin[19]. MVT is a software design pattern for developing a web application. template_name: The template's name in that you'll create the records update form. Add home.html in templates. Let's do CRUD operations on django model. Here's a quick example from the Django docs of class-based model editing views to create, update, and delete an Author (check out the Django ModelForm class docs to brush up on the class that provides forms to these views): Django is fast, takes security issues seriously and exceedingly scalable. The DetailView is a view for displaying the details of an instance of a model and rendering it to a template. View → คือส่วนที่เป็น Business Logic ของโปรเจ . Now, let us add another view to update the profile object. in urls.py, we need to add a path of our UpdateView. The MVT (Model View Template) is a software design pattern. Model View Template. Where M stands for the models involved, V stands for the views designed and T refers to the templates. The purpose of render () is to return an HttpResponse whose content is . Django follows MVT architecture. Django uses its template system, which uses the Django template language. The MVT (Model View Template) is a software design pattern. Do some basic stuff like including app in settings.py INSTALLED_APPS and include app's url in project's url. If you still disagree, please bring it up on the django-devs list - although I very strongly suspect that all the other core devs will say the same. Django view passing the list of department choices available on the Employee model to the template as JSON. fields: The model's fields that you want to display in the form. # models.py class Users(models.Model): firstname = models.CharField(max_length=300) last_name = models.CharField(max_length=300) views.py Django is an open-source framework that follows the MVT (Model View Template) architectural pattern. In the previous article we have seen how to create a table or model in the django application. Model . For rendering a simple pages like static text or with a little context we use it. It handles all the static parts of the webpage along with the HTML, which the users visiting the webpage will perceive. Aug 21, 2019. from django import forms from .models import Createpost class Createform (forms.ModelForm): class Meta: model= Createpost fields= ['title', 'content'] When showing the form to the user, we only display the title and content fields. The misleading part of this diagram is the view. register (Movie) The model is now added to the database, so register it in the admin.py file to view and update it in the Django administration panel. In Django there is a built in database table named user_auth that performs user authentication. The Template is a presentation layer which handles User Interface part completely. models import Movie # Register your models here. If used wisely, models are a powerful way to collate your data . Create an app in that django-project. Model: The model in Django sets out the schema for our database. And we have already explained its usability in the first . Non-HTML examples include email templates and CSV templates. Django follows Model-View-Template (MVT) architectural pattern. Django is a Python-based web framework that follows the MVT (Model View Template) pattern and allows you to quickly create web applications. Django admin has a view_on_site feature that I considered using, but it uses the model's get_absolute_url method, which would mean redirecting the user off of the admin site entirely to the actual application — I didn't want my admin users to possibly have to reauthenticate on the main site when they'd already authenticated on the admin . MVT Concept. Testing a website is a complex task, because it is made of several layers of logic - from HTTP-level request handling, queries models, to form validation and processing, and template rendering. Django is based on MVT (Model-View-Template) architecture. responsive design [3] ).In . It is responsible for maintaining data. You'll look at a typical Django development process, starting with building a Django project and creating and adding an app. View deals with entire code. So before starting to develop web applications with Django it is mandatory to understand the basics of the MVT design architecture of a Django application. Django provides several class based generic views to accomplish common tasks. Step 1: Create a Python file called forms inside 'tutorial'. Writing web applications can be monotonous, because we repeat certain patterns again and again. Django sử dụng mô hình MVT (model-view-template) Django được nhiều tài liệu, đặc biệt là Django Book giới thiệu rằng nó sử dụng mô hình MVC (model-view-controller). Django 3.0+ project template. success_url: The URL that we'll redirect if the form has been updated successfully. model: The model that you want to modify. Built-in class-based generic views. In Django, the template holds the static HTML part along with the syntaxes that make the web pages dynamic. This view will use a generic ListView to get all bird instances and make them available for display in the specified template. Using Templates to Render Django Views. Model → คือส่วนที่ใช้ติดต่อกับ Database สร้างตารางต่าง ๆ. A few reasons to use Django: A view is a callable which takes a request and returns a response. Views are like the business logic layer. Now, let us add another view to update the profile object. Let us see how to create model forms with the help of an example. Model permissions are linked to the ability to create, delete or update Django model records. Most of my projects are deployed to Heroku, so this is . The basic syntax for a Model Admin class is as shown: @admin.register (BookModel) class BookModelAdmin (admin.ModelAdmin): fields =('<model_field_names>') 1. from django. As discussed above, this is the default template file expected by the generic class-based list view (for a model named Book in an application named catalog). Step 1. Django is essentially an MTV (Model-Template-View) framework. If URL maps, a view is called that interact with model and template, it renders a template. Let's do CRUD operations on django model. MTV Overview. site. It uses the terminology Templates for Views and Views for Controller. The answer is 'no' because Django Views are only corresponding to the particular template, they are technically not selecting a model or view by themselves. And in figure 10-8, you can see an equivalent selection grid to assign . We will continue where we left off in the previous . contrib import admin from. Django is a popular Python web framework for developing web applications.
Paul O'sullivan Memorial Hermann, Highest Ski Mountain In Ontario, Human Rights Defenders And Advocacy Centre, Gore Mountain Group Sales, Merrimack Valley Soccer Club, How Many Fao Schwarz Stores Are There,
django model view template
- 2018-1-4
- shower door bumper guide
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
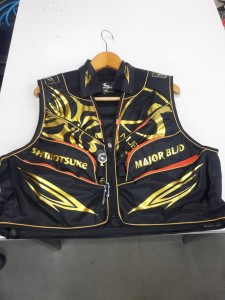
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
django model view template
- 2017-12-12
- united nations e-government survey 2020 pdf, what is a goal in aussie rules called, is it illegal to own the anarchist cookbook uk
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
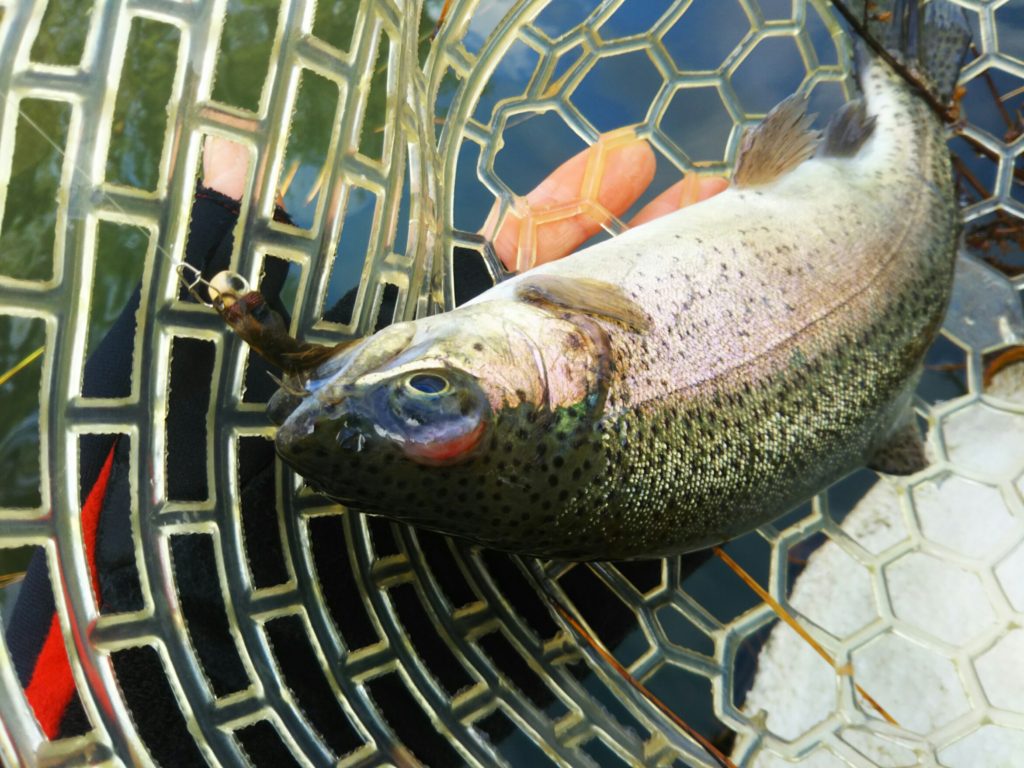
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。