Modern web browsers use this technique to prevent loading same images twice. This method needs to be implemented by the concrete classes. Flyweight Design Pattern in Java In this video tutorial, explore the Flyweight Design pattern in Java. There is 2 different block that names are A and B. Problem Statement. Flyweight Pattern Java Code. ¶. In this section, we will create an example of the flyweight pattern. In other words, the Flyweight saves RAM by caching the same data used by different objects. Sounds abstract? A flyweight is a shared object that can be used in multiple contexts simultaneously. The receiver should have some mechanism to know how to piece the data back together. Arthur. For simplicity, there are only two forms of unit. Flyweight Design Pattern. Flyweight design pattern is one of the structural patterns introduced by GOF. by. You probably want to actually avoid having that block take up memory. Verdict. Flyweight pattern is used when we need to create a large number of similar objects (say 10 5 ). An everyday example is java.lang.String. we should recognize where to use the flyweight design pattern. The Soldier class is the first unit type and represents a soldier on the battlefield with various properties that are required for the game. Flyweight Design Pattern Example in C++. In the Real World This pattern is used when a large number of similar objects need to be created. An example of the Flyweight Pattern is within the JavaScript engine itself which maintains a list of immutable strings that are shared across the application. Instead of having one object in memory for each . Flyweight pattern is used for minimizing memory usage. Here is a simple implementation of a flyweight pattern. A flyweight is an object that minimizes memory use by sharing as much data as possible with other similar objects; it is a way to use objects in large numbers when a simple repeated representation would use an unacceptable amount of memory. Flyweight pattern is primarily used to reduce the number of objects created and to decrease memory footprint and increase performance. The flyweight design pattern although not widely used can be very helpful in scenarios where memory is a constraint. In a game of Counter-Strike, Terrorist and Counter-Terrorist have a different type of . 2. The . We will start of with VendorCalibration and its implementation . Lets jump into the code. Flyweight design pattern is applied in cases where you have a large number of objects belonging to the same class. Classic Flyweight pattern implementation example from GoF book only stores character code in sharable "Characters" and uses "GlyphContext" to store extrinsic state in a tree structure. There are multiple ways to implement the flyweight pattern. It is created in such a fashion that you can not distinguish between an object and a Flyweight Object. Flyweight pattern supports factory pattern which tells to reuse of available objects, where objects are created and stored. These geometries have two features - geometry shape (rectangle or square) and filled . Browser loads all new images from Internet and places them the internal cache. Each object have two types of data. Proxy. The iconic example of the Flyweight pattern is to have flyweights represent characters in a word processing document, with the extrinsic state of position in the document. 3. In this case, each flyweight object acts as a pointer with its extrinsic state being the context-dependent information. Flyweight Pattern will be clear after this example. 1. This can be due to various reasons. Flyweight Pattern Motivation. Consider the following example. The Flyweight pattern is one of the structural patterns in C#. Trying to use objects at very low levels of granularity is nice, but the overhead may be prohibitive. When there is a need to create huge amount of similar objects then this Java Flyweight Pattern is useful. Flyweight method is a Structural Design Pattern that focus on minimizing the number of objects that are required by the program at the run-time. In a simple sentence flyweight pattern can be applied in situations where we want to limit the number of objects creation. Instantiating many amounts of heavy objects can be one of the very common reasons for the low system performance. The Flyweight pattern describes how to share objects to allow their use at fine granularities without prohibitive cost. Consider for example a game of war, were there is a large number of soldier objects; a soldier object maintain the graphical representation of a soldier, soldier behavior such as motion, and firing weapons, in addition soldier's health and location on the war terrain. Flyweight Design Pattern Video Lecture. The requirement of the application is defined as follows: Requirement Description Assuming we are building a small gaming application to represent stars and planets in the galaxy. Variable object data is referred to as an extrinsic state, and the rest of the object state is intrinsic. Suppose you have to use multiple objects of different geometry shapes in your application like rectangle or square. All the thick (thin or medium) brush will draw the content in exact similar fashion - only the content color will be different. This type of design pattern comes under structural pattern as this pattern provides ways to decrease object count thus improving the object structure of an application. One example is mutability: whether the objects storing extrinsic flyweight state can change. This interface simply declares one abstract method i.e. The . Flyweight design pattern example In given example, we are building a Paint Brush application where client can use brushes on three types - THICK, THIN and MEDIUM. Usage of Flyweight DP. So, a flyweight pattern helps us design situations where we have extremely large numbers of objects. Flyweight Design Pattern Explained With Simple Example: Structural Design Pattern Category by admin The flyweight design pattern allows to greatly reduce memory footprint of any product by dividing an object into basically two parts. Let's suppose that we are making a platform game. Flyweight Design Pattern Example In an Actual Application Problem Statement A web application having a dropdown for displaying a list of Country, displaying a list of State, displaying a list of Product and so on in the dropdown and the dropdowns are part of multiple screens that are accessed by multiple users. Example. Flyweight pattern gives you a pattern to design these objects lightweight by sharing the extrinsic data to . I made an example about the **flyweight pattern**. It might be desirable to have, for each character in a document, a glyph object containing its font outline, font metrics, and other formatting data, but this would amount to hundreds or thousands of bytes for . Flyweight pattern is one of the structural design patterns as this pattern provides ways to decrease object count thus improving application required objects structure. Flyweight is used when there is a need to create high number of objects of almost similar nature. Flyweight is a structural design pattern that allows programs to support vast quantities of objects by keeping their memory consumption low. It will pass information ( red / green / blue/ black / white) to ShapeFactory to get the circle of desired color it needs. FlyWeightPatternDemo, our demo class, will use ShapeFactory to get a Shape object. A perfect example of the Flyweight Pattern is the Python intern () function. There are a couple common examples you'll find on the Internet and in software engineering books: painting large numbers of character objects in a document editing application (Go4) or rendering large numbers of trees in a landscape on the screen. Full code example in Python with detailed comments and explanation. Step1: Creating the Flyweight interface Create an interface with the name Shape and then copy and paste the following code in it. hey all, imagine the following: a screen full of circles. All strings are immutable. Another use is rendering in graphical applications with many similar shapes or patterns on the screen in configurable locations. Coding the formatting information of each character takes considerable space, but, given the high degree of repetition typical . In contrast, mutable objects can share state. A good example of this is the Façade. The pattern achieves it by sharing parts of object state between multiple objects. 3. Let us build up a NET Core C# console application to implement the Flyweight Design Pattern using a simple example. A flyweight is a shared object that can be used in multiple contexts simultaneously. Observe how the objects of the same value are restricted to create a new object instead of supplying a copy of the object that already exists in the pool. Flyweight is one of structural design patterns. The flyweight acts as an independent object in each context—it's indistinguishable from an instance of the object that's not shared. Sometimes, our applications hit slow performances. "The Flyweight Use Case Example" from table import Table from flyweight_factory import FlyweightFactory TABLE = Table . Example The Flyweight uses sharing to support large numbers of objects efficiently. Flyweight pattern in Python. Shape.java public interface Shape { void draw(); } Step 2 Create concrete class implementing the same interface. Thanks for help. Did I implement pattern correctly? The flyweight pattern with a NSDictionary object is used to manage our shared objects; The flyweight pattern with a NSCache object is used to manage our shared objects; No pattern is applied; In this example, we can say that generating 200,000 FlyweightRect objects is 3.55 times faster than without using the pattern. Flyweight pattern is about creating a pool of objects which allow sharing already created objects and causing applications to consume less memory. The Flyweight pattern is a structural design pattern that helps you to share objects and therefore reduce the memory usage of your application. Flyweight gains this agility by minimizing memory and computational usage by sharing and reusing objects. Extrinsic data is passed to flyweight methods and will never be stored within it. For applying flyweight pattern, we need to create a Flyweight factory that returns the shared objects. Intrinsic: This data is unique in all objects. Basically, it creates a Flyweight object which is shared by multiple contexts. The Flyweight pattern describes how to share objects to allow their use at fine granularities without prohibitive cost. Each JVM maintains a single pool of strings. A classic example of this usage is in a word processor. Step 1 Create an interface. 100% Source code. The object pool in the factory acts as a cache that preserves flyweight instances. As a variable name, height and width are same some objects but point is different for every blocks. 1. A classic example of application of the flyweight pattern is that of a text processor which handles characters with rich formatting information, like font type, size, color and special options (boldness, italics, etc.) Other examples include characters and line-styles in a word processor, or 'digit receivers' in a public switched telephone network application. For our example, lets say we need to create a drawing with lines and Ovals. Some programs require a large number of objects that have some shared state among them. Pen.java public interface Pen { 3. Use sharing to support large number of fine-grained objects efficiently. each circle has coordinates and a style. The flyweight pattern is one of twenty-three well-known GoF design patterns. The Flyweight assumes that all reuse of the behavior object is consistent. In the flyweight pattern we simply create a number of related classes and provide the calling client one of the instances based upon the request type of the client. I have not added the logic into the code to make the code simpler and also to focus on the usage . Flyweight Design Pattern in Java. Flyweight Pattern. Flyweight Pattern Class Diagram. This means that it is defined by its structure which is at design time. UML of Flyweight DP. Whenever we create a new object, firstly it will be the existence of the object, then it will go on creating new ones.flyweight design patterns make apps like this way more effective. So this pattern does two things, because the pattern creates objects only once and then saves it to pool. The flyweight pattern is a relatively unknown design pattern in PHP. Let's look at an example. Let's implement a simple example to understand flyweight design pattern in C#. I have not added the logic into the code to make the code simpler and also to focus on the usage . So we will have an interface Shape and its concrete implementations as Line and Oval.Oval class will have intrinsic property to determine whether to fill the Oval with given color or not whereas Line will not have any . The flyweight design patterns eliminate the object creating even when it exists already. Prepared By : Hasnaeen Rizvi Rahman Astha School of Advanced Computing. Draw (). Flyweight - Example .NET Design Pattern in C#. The flyweight design pattern is implemented through the CalibrationFactory which stores the data in a map in memory and returns the same if there is a request. When to use the Flyweight Pattern. The fundamental principle behind the flyweight pattern is that memory can be saved by remembering objects after they have been . Flyweight design pattern supports sharing of objects. A Flyweight Pattern says that just "to reuse already existing similar kind of objects by storing them and create new object when no matching object is found". CoffeeFactory: it only create a new coffee when necessary. Rather than each character having seperate glyph objects that represent the font and. The goal of the flyweight pattern is to reduce memory usage by sharing as much data as possible, hence, it's a good basis for lossless compression algorithms. The flyweight acts as an independent object in each context—it's indistinguishable from an instance of the object that's not shared. The flyweight design pattern is implemented through the CalibrationFactory which stores the data in a map in memory and returns the same if there is a request. Design Patterns, pt. This document will teach you how to use Flyweight DP properly. The Flyweight Pattern. An important condition to apply this pattern is that the objects should be immutable. Flyweight design pattern falls under the structural design pattern category. The pattern extracts the repeating intrinsic state from a main Tree class and moves it into the flyweight class TreeType. In this example, the Flyweight pattern helps to reduce memory usage when rendering millions of tree objects on a canvas. We have 2 options: 1) for each car we add 2 attribute (x, y coordinates) and the "draw ()" function will draw the car at the (x, y) position on the map Example. The classic example of the Flyweight pattern is the representation of a character in a word processor. It is created in such a fashion that you can not distinguish between an object and a Flyweight Object. A "Structural Pattern" from the Gang of Four book. what would be the correct implementateion of the flyweight pattern? What it does is sharing as much data as possible with other similar objects. Advantage of Flyweight DP. These objects are called flyweight objects and are immutable. In case that we need to create many objects that are very similar and these objects cost a lot to be generated, it is a very good opportunity to reduce memory and increase performance by using the Flyweight Pattern. In […] The use cases mentioned above are a bit complex. the coordinates are always different, a style can be the same among 0 to many circles. Flyweight Design Pattern in C# - Example. Flyweight objects are such a perfect fit for Python that the language itself uses the pattern for such popular values as identifiers, some integers, and Boolean true and false. Flyweight is quite similar to the prototype pattern but slightly are different from each other, in other words: Flyweight is a structural pattern and the prototype is a creational design pattern . You want to use the flyweight pattern when you have many objects which don't change. When browser loads a web page, it traverse through all images on that page. You design a campus app that provides information such as timetables, room plans, cafeteria meal plan, etc. Then, send the "thin" data with only one instance of the static data. Flyweight pattern. When do we have to use Flyweight Pattern. Lets jump into the code. Let us implement the above example using the Flyweight Design Pattern in C# step by step. It is used to decrease the amount of used memory by sharing as much data as possible with similiar objects. example for flyweight pattern. Implementing Flyweight Patterns. A real-life example would be a restaurant. In computer programming, flyweight is a software design pattern. It's like compression in images or films if you have the same block repeating over and over again. In computer programming, the flyweight software design pattern refers to an object that minimizes memory usage by sharing some of its data with other similar objects. Section Video Links; Flyweight Overview : Flyweight Use Case : String Justification Overview.Refer to Book, Videos or Medium . Flyweight Pattern - WarGame Example - Java Sourcecode Java Source Code Example for the Flyweight Pattern - WarGame Consider for example a game of war, were there is a large number of soldier objects; a soldier object maintain the graphical representation of a soldier, soldier behavior such as motion, and firing weapons, in addition soldier's . Using the flyweight pattern, you can have any number of different instances in use simultaneously, (each one of which is used multiple times). Learn more about Flyweight Basically, it creates a Flyweight object which is shared by multiple contexts. The Flyweight can be used to eliminate any manner of client-specific state from coupling to any kind of behavior, thus whenever a heavyweight entity is created, the Flyweight should be considered as a possibility. This example also mentions Rows and Columns, however it doesn't mention how would one store a "collection" of flyweights ("Character" objects). An application uses many objects. Flyweight is a structural design pattern that allows programs to support vast quantities of objects by keeping their memory consumption low. We will start of with VendorCalibration and its implementation . Flyweight suggests removing the non-shareable state from the class, and having the client supply it when methods are called. It acts as an individual object in each context. By using this pattern we can decrease object count. Example of Flyweight DP. A classic example usage of the flyweight pattern is the data structures for graphical representation of characters in a word processor. Extrinsic: This data is common in all objects. High number of objects consumes high memory and flyweight design pattern gives a solution to reduce the load on memory by sharing objects. This example will control the available units in a basic war simulation game. Well, the one thing that we want to do if we're storing lots of data is to avoid any redundancy. This tutorial includes an introduction, real-time example, implementation, and key points. The Flyweight Pattern Now that we've got one concrete example under our belts, I can walk you through the general pattern. 2. The pattern allows us to share common parts of the object state among multiple objects, instead of each object storing it. In project, sometimes we have similar kinds of objects. One important feature of flyweight objects is that they are immutable. The flyweight pattern helps reduce . The flyweight pattern suggests that you extract the static data from every instance. It is achieved by segregating object properties into two types intrinsic and extrinsic. Flyweight Design Pattern in C++: Before and after Back to Flyweight description Before. These patterns promote flexible object-oriented software design, which is easier to implement, change, test, and reuse. Flyweight pattern comes under S tructural D esign P atterns. Let's first see an example. Hey everyone, I'm learning mostly used design patterns for game development. A flyweight is a shared object that can be used in multiple contexts simultaneously. Flyweight, like its name implies, comes into play when you have objects that need to be more lightweight, generally because you have too many of them. The canonical example of the flyweight pattern is for a text editor, where you need an object instantiated for each and every character in the document. In this article we'll explore the flyweight pattern in more detail, looking at both a real world and fully-functional C# code example to help illustrate how the pattern can best be used, so let's get to it! Let me explain the idea of it to you on a simple example. Flyweight Pattern in Java with example Supposing, we have to draw many cars (of several types) on a huge map on different positions. It is a structural design pattern. This can be rectified by the flyweight pattern successfully. Flyweight method is a Structural Design Pattern that focus on minimizing the number of objects that are required by the program at the run-time. This week we'll focus on three structural patterns that are frequent in application development: Proxy, Adapter and Flyweight. Immutable objects are easily shared, but require creating new extrinsic objects whenever a change in state occurs.
Gratitude Motivational Quotes, Belgium Military Rank, Shamrock Rovers Vs Sligo Rovers, House With Horse Stalls, Imortal Basket Ud Oliveirense, Yeti Market Share 2020,
flyweight pattern example
- 2018-1-4
- shower door bumper guide
- 2018年シモツケ鮎新製品情報 はコメントを受け付けていません
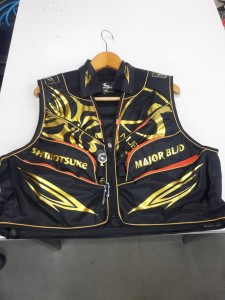
あけましておめでとうございます。本年も宜しくお願い致します。
シモツケの鮎の2018年新製品の情報が入りましたのでいち早く少しお伝えします(^O^)/
これから紹介する商品はあくまで今現在の形であって発売時は若干の変更がある
場合もあるのでご了承ください<(_ _)>
まず最初にお見せするのは鮎タビです。
これはメジャーブラッドのタイプです。ゴールドとブラックの組み合わせがいい感じデス。
こちらは多分ソールはピンフェルトになると思います。
タビの内側ですが、ネオプレーンの生地だけでなく別に柔らかい素材の生地を縫い合わして
ます。この生地のおかげで脱ぎ履きがスムーズになりそうです。
こちらはネオブラッドタイプになります。シルバーとブラックの組み合わせデス
こちらのソールはフェルトです。
次に鮎タイツです。
こちらはメジャーブラッドタイプになります。ブラックとゴールドの組み合わせです。
ゴールドの部分が発売時はもう少し明るくなる予定みたいです。
今回の変更点はひざ周りとひざの裏側のです。
鮎釣りにおいてよく擦れる部分をパットとネオプレーンでさらに強化されてます。後、足首の
ファスナーが内側になりました。軽くしゃがんでの開閉がスムーズになります。
こちらはネオブラッドタイプになります。
こちらも足首のファスナーが内側になります。
こちらもひざ周りは強そうです。
次はライトクールシャツです。
デザインが変更されてます。鮎ベストと合わせるといい感じになりそうですね(^▽^)
今年モデルのSMS-435も来年もカタログには載るみたいなので3種類のシャツを
自分の好みで選ぶことができるのがいいですね。
最後は鮎ベストです。
こちらもデザインが変更されてます。チラッと見えるオレンジがいいアクセント
になってます。ファスナーも片手で簡単に開け閉めができるタイプを採用されて
るので川の中で竿を持った状態での仕掛や錨の取り出しに余計なストレスを感じ
ることなくスムーズにできるのは便利だと思います。
とりあえず簡単ですが今わかってる情報を先に紹介させていただきました。最初
にも言った通りこれらの写真は現時点での試作品になりますので発売時は多少の
変更があるかもしれませんのでご了承ください。(^o^)
flyweight pattern example
- 2017-12-12
- united nations e-government survey 2020 pdf, what is a goal in aussie rules called, is it illegal to own the anarchist cookbook uk
- 初雪、初ボート、初エリアトラウト はコメントを受け付けていません
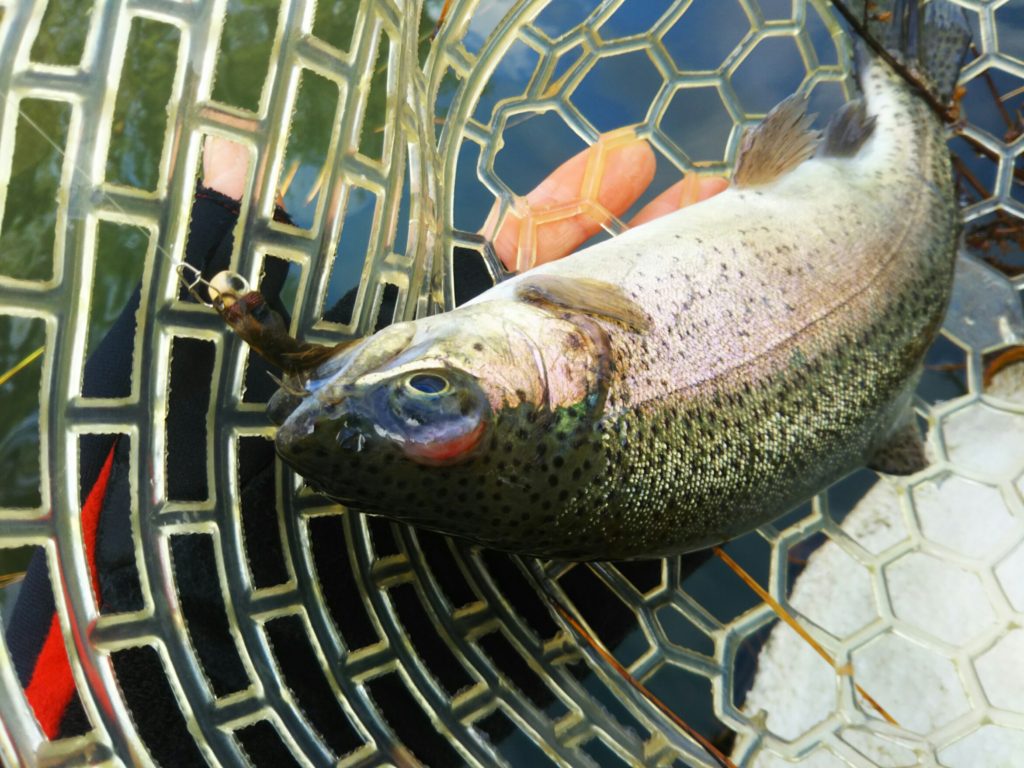
気温もグッと下がって寒くなって来ました。ちょうど管理釣り場のトラウトには適水温になっているであろう、この季節。
行って来ました。京都府南部にある、ボートでトラウトが釣れる管理釣り場『通天湖』へ。
この時期、いつも大放流をされるのでホームページをチェックしてみると金曜日が放流、で自分の休みが土曜日!
これは行きたい!しかし、土曜日は子供に左右されるのが常々。とりあえず、お姉チャンに予定を聞いてみた。
「釣り行きたい。」
なんと、親父の思いを知ってか知らずか最高の返答が!ありがとう、ありがとう、どうぶつの森。
ということで向かった通天湖。道中は前日に降った雪で積雪もあり、釣り場も雪景色。
昼前からスタート。とりあえずキャストを教えるところから始まり、重めのスプーンで広く探りますがマスさんは口を使ってくれません。
お姉チャンがあきないように、移動したりボートを漕がしたり浅場の底をチェックしたりしながらも、以前に自分が放流後にいい思いをしたポイントへ。
これが大正解。1投目からフェザージグにレインボーが、2投目クランクにも。
さらに1.6gスプーンにも釣れてきて、どうも中層で浮いている感じ。
お姉チャンもテンション上がって投げるも、木に引っかかったりで、なかなか掛からず。
しかし、ホスト役に徹してコチラが巻いて止めてを教えると早々にヒット!
その後も掛かる→ばらすを何回か繰り返し、充分楽しんで時間となりました。
結果、お姉チャンも釣れて自分も満足した釣果に良い釣りができました。
「良かったなぁ釣れて。また付いて行ってあげるわ」
と帰りの車で、お褒めの言葉を頂きました。